3、编写GUI界面,模拟抢红包游戏,在界面输入红包大小和抢红包人姓名(用逗号隔开),输出系统当前时间以及每个人抢的红包大小,要求每个人抢的红包最少不能少于0.01元,精确到小数点后两位。
时间: 2024-03-31 18:36:42 浏览: 27
好的,这个问题需要用到Python的tkinter模块来实现GUI界面,以下是代码实现:
```python
import tkinter as tk
from datetime import datetime
class RedEnvelopeGame:
def __init__(self, window):
self.window = window
self.window.title("抢红包游戏")
self.window.geometry('400x300')
self.label1 = tk.Label(window, text="红包大小(元):")
self.label1.pack()
self.entry1 = tk.Entry(window)
self.entry1.pack()
self.label2 = tk.Label(window, text="抢红包人姓名(用逗号隔开):")
self.label2.pack()
self.entry2 = tk.Entry(window)
self.entry2.pack()
self.button = tk.Button(window, text="抢红包", command=self.click_button)
self.button.pack()
self.text = tk.Text(window)
self.text.pack()
def click_button(self):
money = float(self.entry1.get()) # 获取红包大小
names = self.entry2.get().split(",") # 获取抢红包人姓名列表
time = datetime.now().strftime("%Y-%m-%d %H:%M:%S") # 获取当前时间
# 计算每个人抢到的红包金额
total_money = 0
for i in range(len(names)):
if i == len(names) - 1:
money_i = round(money - total_money, 2)
else:
money_i = round(money * (1 / len(names)), 2)
money_i = max(money_i, 0.01)
total_money += money_i
self.text.insert('end', f"{names[i]}抢到了{money_i}元\n")
self.text.insert('end', f"系统当前时间:{time}\n\n")
window = tk.Tk()
game = RedEnvelopeGame(window)
window.mainloop()
```
运行代码后,会弹出一个窗口,输入红包大小和抢红包人姓名后,点击“抢红包”按钮,就会在窗口下方输出每个人抢到的红包金额和系统当前时间。注意,代码中做了一些限制,每个人抢到的红包金额最少为0.01元,且最后一个人抢到的金额为总金额减去前面所有人抢到的金额之和。
相关推荐
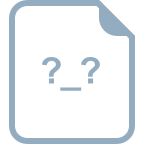
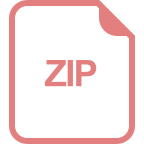
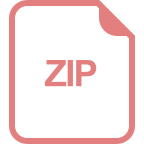
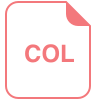
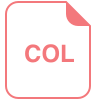









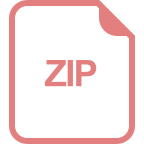
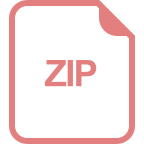
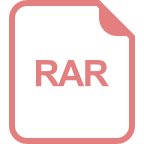