@Override public void addAdvert(AdvertDTO advertDto) { //MediaFile mediaFile = new MediaFile(); AdPosition adPosition = Optional.ofNullable(adPositionMapper.selectByPrimaryKey(advertDto.getPositionId())) .orElseThrow(() -> new RuntimeException("没有找到对应的广告位置")); Advert advert = Advert.builder() .name(advertDto.getName()) .position(adPosition) .media(advertDto.getMediaFile()) .startDate(advertDto.getStartDate()) .endDate(advertDto.getEndDate()) .link(advertDto.getLink()) .status(advertDto.getStatus()) .build(); AdvertMapper.insertSelective(advert); } @Override public void updateAdvert(Integer id, AdvertDTO advertDto) { AdPosition adPosition = Optional.ofNullable(adPositionMapper.selectByPrimaryKey(advertDto.getPositionId())) .orElseThrow(() -> new RuntimeException("Ad position not found")); Advert advert = Advert.builder() .name(advertDto.getName()) .position(adPosition) .media(advertDto.getMediaFile()) .startDate(advertDto.getStartDate()) .endDate(advertDto.getEndDate()) .link(advertDto.getLink()) .status(null != advertDto.getStatus() ? advertDto.getStatus() : 0) .build(); AdvertMapper.insert(advert); },如何优化代码
时间: 2024-02-14 08:05:20 浏览: 114
首先需要注意的是,在 updateAdvert 方法中,我们应该使用 updateByPrimaryKeySelective 方法而不是 insert 方法,因为我们只需要更新部分字段而不是全部字段。
另外,在两个方法中,我们可以使用 AdvertMapper 的自动生成代码中的 updateByExampleSelective 方法和 insertSelective 方法,这样可以减少手动编写 SQL 语句的工作量,也可以更简洁地实现相同的功能。
最后,我们可以将 Optional.ofNullable().orElseThrow() 的代码块提取出来作为一个方法,这样可以使代码更加简洁易读,也可以提高代码的复用性。
综上所述,下面是优化后的代码示例:
```
private AdPosition getAdPositionById(Integer positionId) {
return Optional.ofNullable(adPositionMapper.selectByPrimaryKey(positionId))
.orElseThrow(() -> new RuntimeException("Ad position not found"));
}
@Override
public void addAdvert(AdvertDTO advertDto) {
Advert advert = Advert.builder()
.name(advertDto.getName())
.position(getAdPositionById(advertDto.getPositionId()))
.media(advertDto.getMediaFile())
.startDate(advertDto.getStartDate())
.endDate(advertDto.getEndDate())
.link(advertDto.getLink())
.status(advertDto.getStatus())
.build();
advertMapper.insertSelective(advert);
}
@Override
public void updateAdvert(Integer id, AdvertDTO advertDto) {
Advert advert = Advert.builder()
.name(advertDto.getName())
.position(getAdPositionById(advertDto.getPositionId()))
.media(advertDto.getMediaFile())
.startDate(advertDto.getStartDate())
.endDate(advertDto.getEndDate())
.link(advertDto.getLink())
.status(null != advertDto.getStatus() ? advertDto.getStatus() : 0)
.build();
AdvertExample example = new AdvertExample();
example.createCriteria().andIdEqualTo(id);
advertMapper.updateByExampleSelective(advert, example);
}
```
阅读全文
相关推荐
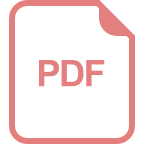
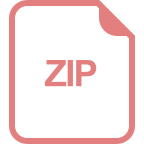
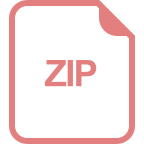
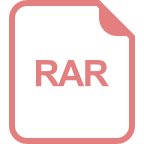
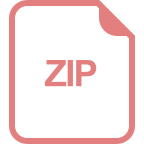
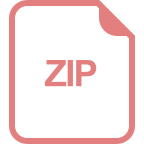
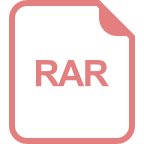
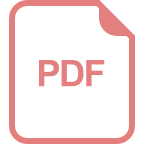
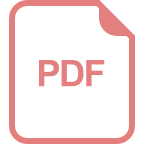
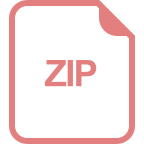
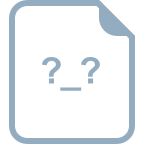
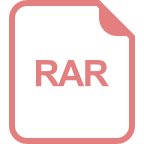
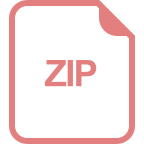
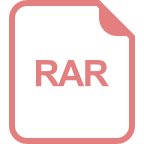
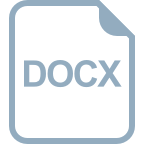
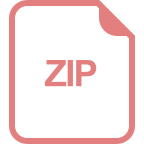
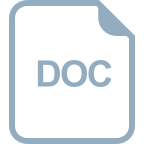
