能补充这段代码吗import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.Pane; import javafx.scene.paint.Color; import javafx.scene.shape.Circle; import javafx.scene.shape.Rectangle; import javafx.scene.shape.Polygon; import javafx.stage.Stage; import java.io.FileInputStream; import java.io.IOException; import java.util.Properties; abstract class Shape { protected Color color; protected double area; protected double perimeter; protected double positionX; protected double positionY; public abstract void calculateArea(); public abstract void calculatePerimeter(); public abstract void draw(Pane pane); public void setPosition(double x, double y) { this.positionX = x; this.positionY = y; } } class CircleShape extends Shape { private double radius; public CircleShape(double radius) { this.radius = radius; this.color = Color.RED; } @Override public void calculateArea() { this.area = Math.PI * Math.pow(radius, 2); } @Override public void calculatePerimeter() { this.perimeter = 2 * Math.PI * radius; } @Override public void draw(Pane pane) { Circle circle = new Circle(radius); circle.setFill(color); circle.setLayoutX(positionX); circle.setLayoutY(positionY); pane.getChildren().add(circle); } } class RectangleShape extends Shape { private double width; private double height; public RectangleShape(double width, double height) { this.width = width; this.height = height; this.color = Color.BLUE; } @Override public void calculateArea() { this.area = width * height; } @Override public void calculatePerimeter() { this.perimeter = 2 * (width + height); } @Override public void draw(Pane pane) { Rectangle rectangle = new Rectangle(width, height); rectangle.setFill(color); rectangle.setLayoutX(positionX); rectangle.setLayoutY(positionY); pane.getChildren().add(rectangle); } } class TriangleShape extends Shape { private double[] points; public TriangleShape(double[] points) { this.points = points; this.color = Color.GREEN; } @Override public void calculateArea() { double x1 = points[0]; double y1 = points[1]; doubl
时间: 2023-12-06 19:41:17 浏览: 101
e x2 = points[2]; double y2 = points[3]; double x3 = points[4]; double y3 = points[5]; this.area = Math.abs((x1 * (y2 - y3) + x2 * (y3 - y1) + x3 * (y1 - y2)) / 2.0); } @Override public void calculatePerimeter() { double side1 = Math.sqrt(Math.pow(points[0] - points[2], 2) + Math.pow(points[1] - points[3], 2)); double side2 = Math.sqrt(Math.pow(points[2] - points[4], 2) + Math.pow(points[3] - points[5], 2)); double side3 = Math.sqrt(Math.pow(points[4] - points[0], 2) + Math.pow(points[5] - points[1], 2)); this.perimeter = side1 + side2 + side3; } @Override public void draw(Pane pane) { Polygon triangle = new Polygon(points); triangle.setFill(color); triangle.setLayoutX(positionX); triangle.setLayoutY(positionY); pane.getChildren().add(triangle); } } public class Main extends Application { @Override public void start(Stage primaryStage) throws Exception { Pane pane = new Pane(); Properties prop = new Properties(); try { FileInputStream input = new FileInputStream("config.properties"); prop.load(input); input.close(); } catch (IOException ex) { ex.printStackTrace(); } for (int i = 1; i <= 3; i++) { String shapeType = prop.getProperty("shape" + i + ".type"); Shape shape; if (shapeType.equals("circle")) { double radius = Double.parseDouble(prop.getProperty("shape" + i + ".radius")); shape = new CircleShape(radius); } else if (shapeType.equals("rectangle")) { double width = Double.parseDouble(prop.getProperty("shape" + i + ".width")); double height = Double.parseDouble(prop.getProperty("shape" + i + ".height")); shape = new RectangleShape(width, height); } else { double[] points = new double[6]; for (int j = 0; j < 6; j++) { points[j] = Double.parseDouble(prop.getProperty("shape" + i + ".point" + (j+1))); } shape = new TriangleShape(points); } shape.calculateArea(); shape.calculatePerimeter(); double posX = Double.parseDouble(prop.getProperty("shape" + i + ".posX")); double posY = Double.parseDouble(prop.getProperty("shape" + i + ".posY")); shape.setPosition(posX, posY); shape.draw(pane); } Scene scene = new Scene(pane, 500, 500); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
这段代码实现了一个基于JavaFX的图形绘制应用,它定义了一个抽象类 Shape 和三个子类 CircleShape、RectangleShape 和 TriangleShape,分别对应圆形、矩形和三角形,每个子类都实现了抽象方法 calculateArea()、calculatePerimeter() 和 draw()。这些方法用于计算每个图形的面积、周长和绘制图形。在主类 Main 中,从配置文件 config.properties 中读取每个图形的类型、参数和位置,然后创建相应的图形对象并调用其方法进行计算和绘制。最后将绘制结果显示在 JavaFX 的场景中。
阅读全文
相关推荐
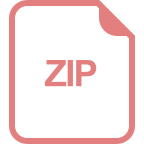
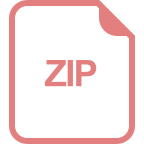
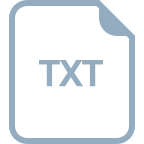











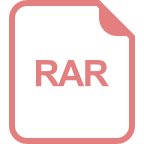
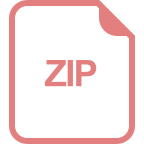



