编写程序实现以下要求: 1.创建Student类存储每个学生信息,属性(学号,姓名,出生日期,得分) 2.从c:/test/student.txt文件中读取学生信息。如下: 学号,姓名,出生日期,得分 1,张三,1982-1-1,80 2,李四,1982-11-15,40 3,王五,1982-2-8,60 4,赵六,1982-7-5,70 3.使用List存储6名学生的信息。 4.使用集合排序,将学生信息按时得分从高到低排序,得分相同时按照出生日期升序排序。 5.输出排序后的结果到c:/test/result.txt文件中,输出格式与输入格式相同,第一行是表头。 6.在代码中使用泛型,读文本文件使用BufferedReader,写文本文件使用BufferedWriterJAVA代码实现加注释
时间: 2024-02-16 09:04:23 浏览: 88
```
import java.io.*;
import java.util.*;
// 定义Student类
class Student implements Comparable<Student>{
private int id; // 学号
private String name; // 姓名
private Date birthDate; // 出生日期
private int score; // 得分
// 构造函数
public Student(int id, String name, Date birthDate, int score) {
this.id = id;
this.name = name;
this.birthDate = birthDate;
this.score = score;
}
// 实现Comparable接口的compareTo方法,用于排序
public int compareTo(Student o) {
if (this.score != o.score) {
// 分数不相等,按分数从高到低排序
return o.score - this.score;
} else {
// 分数相等,按出生日期升序排序
return this.birthDate.compareTo(o.birthDate);
}
}
// 重写toString方法,用于输出
public String toString() {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
return this.id + "," + this.name + "," + sdf.format(this.birthDate) + "," + this.score;
}
}
public class SortStudents {
public static void main(String[] args) {
// 读取文件并创建Student对象
List<Student> list = new ArrayList<>();
try (BufferedReader br = new BufferedReader(new FileReader("c:/test/student.txt"))) {
String line;
while ((line = br.readLine()) != null) {
String[] arr = line.split(",");
int id = Integer.parseInt(arr[0]);
String name = arr[1];
Date birthDate = new SimpleDateFormat("yyyy-MM-dd").parse(arr[2]);
int score = Integer.parseInt(arr[3]);
list.add(new Student(id, name, birthDate, score));
}
} catch (IOException | ParseException e) {
e.printStackTrace();
}
// 排序
Collections.sort(list);
// 输出到文件
try (BufferedWriter bw = new BufferedWriter(new FileWriter("c:/test/result.txt"))) {
bw.write("学号,姓名,出生日期,得分\n");
for (Student stu : list) {
bw.write(stu.toString() + "\n");
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
注释已经写在代码中,主要是定义了一个`Student`类,实现了`Comparable`接口的`compareTo`方法用于排序,然后读取文件并创建`Student`对象,使用`Collections.sort`方法排序,最后输出到文件中。使用了`try-with-resources`语句,可以自动关闭资源。
阅读全文
相关推荐
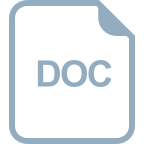
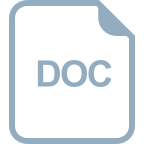
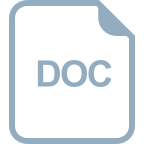

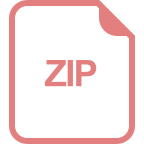

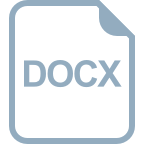
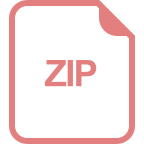
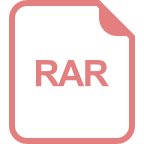
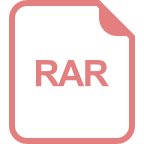
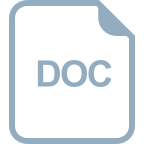
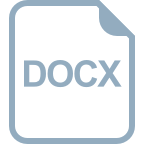
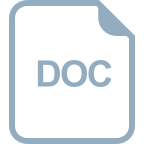
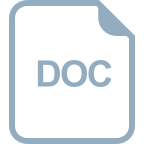
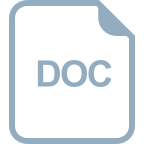
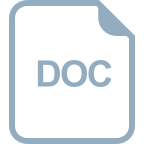
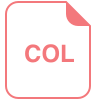

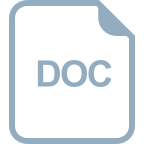