android studio 圆形头像
时间: 2023-09-11 12:03:34 浏览: 263
要在 Android Studio 中实现圆形头像,可以使用以下步骤:
1. 在 xml 布局文件中,添加一个 ImageView 控件,并设置它的宽度和高度相等。
```
<ImageView
android:id="@+id/profile_image"
android:layout_width="100dp"
android:layout_height="100dp" />
```
2. 在 Java 代码中获取 ImageView 控件的引用,并使用 Glide 或其他图片加载库加载图片。
```
ImageView profileImage = findViewById(R.id.profile_image);
Glide.with(this).load(R.drawable.profile_pic).into(profileImage);
```
3. 创建一个圆形裁剪的工具类 CircleTransform,代码如下:
```
public class CircleTransform extends BitmapTransformation {
@Override
protected Bitmap transform(BitmapPool pool, Bitmap toTransform, int outWidth, int outHeight) {
return circleCrop(pool, toTransform);
}
private static Bitmap circleCrop(BitmapPool pool, Bitmap source) {
if (source == null) return null;
int size = Math.min(source.getWidth(), source.getHeight());
int x = (source.getWidth() - size) / 2;
int y = (source.getHeight() - size) / 2;
Bitmap squared = Bitmap.createBitmap(source, x, y, size, size);
Bitmap result = pool.get(size, size, Bitmap.Config.ARGB_8888);
if (result == null) {
result = Bitmap.createBitmap(size, size, Bitmap.Config.ARGB_8888);
}
Canvas canvas = new Canvas(result);
Paint paint = new Paint();
paint.setShader(new BitmapShader(squared, BitmapShader.TileMode.CLAMP, BitmapShader.TileMode.CLAMP));
paint.setAntiAlias(true);
float r = size / 2f;
canvas.drawCircle(r, r, r, paint);
return result;
}
@Override
public void updateDiskCacheKey(@NonNull MessageDigest messageDigest) {
}
}
```
4. 在 Java 代码中使用 CircleTransform 工具类对 ImageView 控件进行圆形裁剪。
```
ImageView profileImage = findViewById(R.id.profile_image);
Glide.with(this).load(R.drawable.profile_pic).transform(new CircleTransform()).into(profileImage);
```
这样就可以在 Android Studio 中实现圆形头像了。
阅读全文
相关推荐
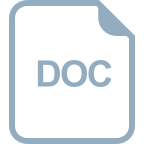
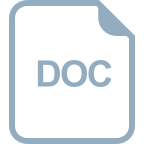
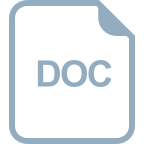


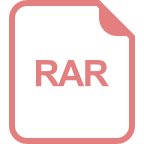

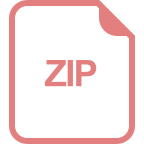
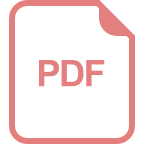
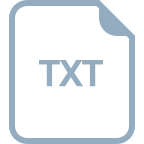
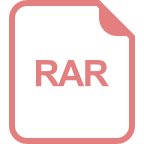
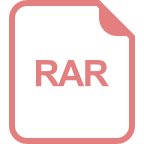
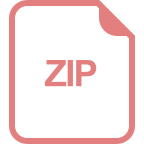




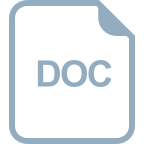
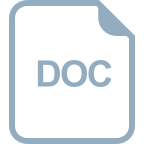