Java Web 中分别使用session,application和文件存储方式实现网站计数器功能,写出代码
时间: 2023-11-10 15:06:35 浏览: 42
使用session实现网站计数器:
在Servlet中:
```java
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
HttpSession session = request.getSession();
Integer count = (Integer) session.getAttribute("count");
if (count == null) {
count = 1;
} else {
count++;
}
session.setAttribute("count", count);
response.getWriter().print("You are the " + count + "th visitor.");
}
```
使用application实现网站计数器:
在Servlet中:
```java
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
ServletContext application = getServletContext();
Integer count = (Integer) application.getAttribute("count");
if (count == null) {
count = 1;
} else {
count++;
}
application.setAttribute("count", count);
response.getWriter().print("You are the " + count + "th visitor.");
}
```
使用文件存储方式实现网站计数器:
```java
public class CounterUtil {
private static final String FILE_NAME = "counter.txt";
public static int getCount() {
int count = 0;
File file = new File(FILE_NAME);
if (file.exists()) {
try (BufferedReader reader = new BufferedReader(new FileReader(file))) {
String line = reader.readLine();
if (line != null) {
count = Integer.parseInt(line.trim());
}
} catch (IOException e) {
e.printStackTrace();
}
}
count++;
try (BufferedWriter writer = new BufferedWriter(new FileWriter(file))) {
writer.write(String.valueOf(count));
} catch (IOException e) {
e.printStackTrace();
}
return count;
}
}
```
在Servlet中:
```java
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int count = CounterUtil.getCount();
response.getWriter().print("You are the " + count + "th visitor.");
}
```
相关推荐
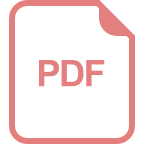
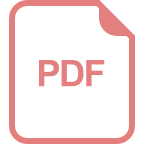
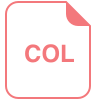
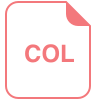












