用python写一个囚徒困境游戏的游戏界面
时间: 2023-12-21 15:05:22 浏览: 37
好的,我可以为您提供一些参考代码。
首先,我们需要安装 `pygame` 库,用于创建游戏界面。可以通过以下命令进行安装:
```
pip install pygame
```
然后,我们可以创建一个游戏类 `PrisonerGame`,包含游戏的初始化、游戏循环、事件处理等方法。具体代码如下:
```python
import pygame
import random
# 设置游戏界面大小
SCREEN_WIDTH = 480
SCREEN_HEIGHT = 480
# 设置颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
class PrisonerGame:
def __init__(self):
pygame.init()
self.screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("囚徒困境游戏")
self.clock = pygame.time.Clock()
self.font = pygame.font.SysFont("Arial", 18)
self.players = ["玩家1", "玩家2"]
self.player_choices = [None, None]
self.scores = [0, 0]
self.round = 1
self.max_rounds = 10
def run(self):
running = True
while running:
self.clock.tick(60)
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_ESCAPE:
running = False
elif event.key == pygame.K_1:
self.player_choices[0] = "B"
elif event.key == pygame.K_2:
self.player_choices[0] = "C"
elif event.key == pygame.K_3:
self.player_choices[1] = "B"
elif event.key == pygame.K_4:
self.player_choices[1] = "C"
if all(self.player_choices):
self.calculate_scores()
self.show_results()
self.next_round()
self.draw()
pygame.display.update()
pygame.quit()
def draw(self):
self.screen.fill(WHITE)
# 绘制分数
text = self.font.render("分数:{} - {}".format(self.scores[0], self.scores[1]), True, BLACK)
self.screen.blit(text, (10, 10))
# 绘制回合数
text = self.font.render("第{}回合 / 共{}回合".format(self.round, self.max_rounds), True, BLACK)
self.screen.blit(text, (10, 30))
# 绘制玩家选择
for i in range(2):
text = self.font.render("{}选择:{}".format(self.players[i], self.player_choices[i] or "-"), True, BLACK)
self.screen.blit(text, (10, 50 + i * 20))
# 绘制提示信息
if not all(self.player_choices):
text = self.font.render("请玩家1按1键选择B,2键选择C;请玩家2按3键选择B,4键选择C", True, BLACK)
self.screen.blit(text, (10, 100))
def calculate_scores(self):
if self.player_choices[0] == "B" and self.player_choices[1] == "B":
self.scores[0] += 1
self.scores[1] += 1
elif self.player_choices[0] == "B" and self.player_choices[1] == "C":
self.scores[0] += 3
elif self.player_choices[0] == "C" and self.player_choices[1] == "B":
self.scores[1] += 3
elif self.player_choices[0] == "C" and self.player_choices[1] == "C":
self.scores[0] += 2
self.scores[1] += 2
def show_results(self):
text = self.font.render("本回合结果:{} - {}".format(self.scores[0], self.scores[1]), True, BLACK)
self.screen.blit(text, (10, 150))
def next_round(self):
self.player_choices = [None, None]
self.round += 1
if self.round > self.max_rounds:
self.show_final_results()
def show_final_results(self):
winner = None
if self.scores[0] > self.scores[1]:
winner = self.players[0]
elif self.scores[0] < self.scores[1]:
winner = self.players[1]
text = self.font.render("比赛结束,{}获胜!".format(winner or "平局"), True, BLACK)
self.screen.blit(text, (10, 200))
if __name__ == "__main__":
game = PrisonerGame()
game.run()
```
这段代码实现了一个简单的囚徒困境游戏界面,玩家通过按键选择合作或背叛,每回合结束后根据双方选择计算得分,最终显示比赛结果。您可以根据自己的需要进行修改和完善。
相关推荐
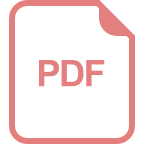
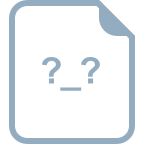














