用python写一个有界面的扫雷游戏
时间: 2023-02-20 08:07:50 浏览: 100
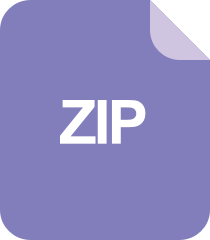
使用Python的扫雷游戏

在 Python 中,您可以使用 Tkinter 库来创建带有图形界面的扫雷游戏。Tkinter 是 Python 内置的 GUI 库,它提供了许多有用的工具来创建窗口、按钮、标签等。
以下是一个基本的例子,该示例实现了一个带有图形界面的扫雷游戏:
```
import tkinter as tk
class Minesweeper(tk.Tk):
def __init__(self, rows, columns, mines):
super().__init__()
self.title("Minesweeper")
self.geometry(f"{rows * 40}x{columns * 40}")
# 这里添加游戏逻辑
if __name__ == "__main__":
game = Minesweeper(10, 10, 10)
game.mainloop()
```
这是一个非常简单的扫雷游戏代码,它仅实现了图形界面。如果要完成游戏逻辑,您可以在 `__init__` 方法中添加额外的代码。
阅读全文
相关推荐
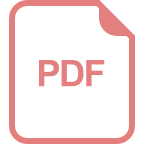














