用python的pygame写一个扫雷游戏,能运行
时间: 2023-06-27 08:05:59 浏览: 152
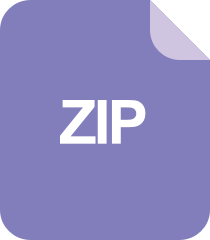
使用Python的扫雷游戏

好的,下面是一个简单的扫雷游戏实现,使用Python的pygame库:
```python
import pygame
import random
# 初始化游戏
pygame.init()
# 设置游戏窗口尺寸
WIDTH = 500
HEIGHT = 600
WINDOW_SIZE = (WIDTH, HEIGHT)
# 设置游戏窗口标题
pygame.display.set_caption("扫雷游戏")
# 定义常用颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GRAY = (128, 128, 128)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
YELLOW = (255, 255, 0)
# 定义游戏区域尺寸和格子数量
GRID_SIZE = 20
GRID_NUM = int(WIDTH / GRID_SIZE)
# 定义雷区和雷数量
mines = []
mine_num = 50
# 创建游戏窗口
screen = pygame.display.set_mode(WINDOW_SIZE)
# 创建字体对象
font = pygame.font.SysFont(None, 40)
# 创建游戏结束标志
game_over = False
# 生成随机雷区
def generate_mines():
global mines
# 随机生成雷的位置
for i in range(mine_num):
mine = (random.randint(0, GRID_NUM - 1), random.randint(0, GRID_NUM - 1))
while mine in mines:
mine = (random.randint(0, GRID_NUM - 1), random.randint(0, GRID_NUM - 1))
mines.append(mine)
# 计算每个格子周围的雷数
def count_mines(x, y):
count = 0
for i in range(-1, 2):
for j in range(-1, 2):
if (x + i, y + j) in mines:
count += 1
return count
# 绘制游戏界面
def draw_game():
global game_over
# 绘制游戏区域
screen.fill(WHITE)
for i in range(GRID_NUM):
for j in range(GRID_NUM):
pygame.draw.rect(screen, GRAY, (i * GRID_SIZE, j * GRID_SIZE, GRID_SIZE, GRID_SIZE), 1)
if (i, j) in mines and not game_over:
pygame.draw.circle(screen, BLACK, (i * GRID_SIZE + GRID_SIZE // 2, j * GRID_SIZE + GRID_SIZE // 2), GRID_SIZE // 4)
elif (i, j) not in mines and not game_over:
count = count_mines(i, j)
if count > 0:
text = font.render(str(count), True, BLUE)
screen.blit(text, (i * GRID_SIZE + GRID_SIZE // 2 - text.get_width() // 2, j * GRID_SIZE + GRID_SIZE // 2 - text.get_height() // 2))
# 绘制游戏结束信息
if game_over:
text = font.render("Game Over", True, RED)
screen.blit(text, (WIDTH // 2 - text.get_width() // 2, HEIGHT // 2 - text.get_height() // 2))
# 刷新屏幕
pygame.display.flip()
# 主循环
generate_mines()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
if not game_over:
x, y = pygame.mouse.get_pos()
i, j = x // GRID_SIZE, y // GRID_SIZE
if (i, j) in mines:
game_over = True
else:
count = count_mines(i, j)
if count == 0:
# 连续翻开空白格子
visited = set()
queue = [(i, j)]
while queue:
x, y = queue.pop(0)
visited.add((x, y))
for m in range(-1, 2):
for n in range(-1, 2):
if 0 <= x + m < GRID_NUM and 0 <= y + n < GRID_NUM and (x + m, y + n) not in visited:
count = count_mines(x + m, y + n)
if count == 0:
queue.append((x + m, y + n))
elif count > 0:
visited.add((x + m, y + n))
for x, y in visited:
if (x, y) not in mines:
count = count_mines(x, y)
if count > 0:
text = font.render(str(count), True, BLUE)
screen.blit(text, (x * GRID_SIZE + GRID_SIZE // 2 - text.get_width() // 2, y * GRID_SIZE + GRID_SIZE // 2 - text.get_height() // 2))
pygame.draw.rect(screen, WHITE, (x * GRID_SIZE, y * GRID_SIZE, GRID_SIZE, GRID_SIZE))
else:
# 翻开数字格子
text = font.render(str(count), True, BLUE)
screen.blit(text, (i * GRID_SIZE + GRID_SIZE // 2 - text.get_width() // 2, j * GRID_SIZE + GRID_SIZE // 2 - text.get_height() // 2))
pygame.draw.rect(screen, WHITE, (i * GRID_SIZE, j * GRID_SIZE, GRID_SIZE, GRID_SIZE))
else:
game_over = False
mines.clear()
generate_mines()
draw_game()
```
这个程序使用了Pygame库来实现,可以直接运行,生成一个简单的扫雷游戏界面。其中,generate_mines()函数用于随机生成雷区,count_mines(x, y)函数用于计算每个格子周围的雷数,draw_game()函数用于绘制游戏界面,主循环监听鼠标事件,根据用户的操作来更新游戏状态和界面。
阅读全文
相关推荐
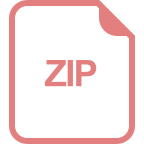
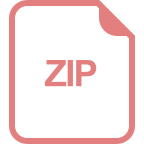




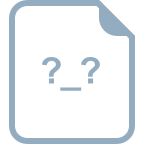

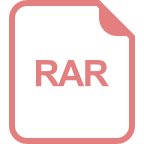
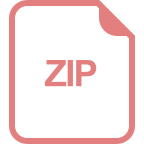
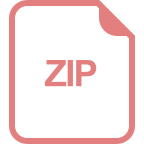
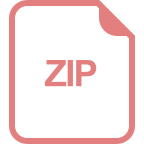
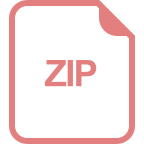
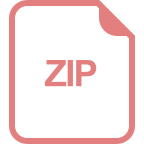


