用python的pygame写一个扫雷游戏,要求能正常游戏
时间: 2023-07-04 21:03:24 浏览: 106
好的,下面是一个简单的扫雷游戏的代码,使用 Python 的 Pygame 库实现:
```python
import pygame
import random
# 游戏配置
WIDTH = 400
HEIGHT = 400
FPS = 30
TILESIZE = 20
BOMBS = 40
# 颜色定义
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GREY = (192, 192, 192)
RED = (255, 0, 0)
# 初始化 Pygame 库
pygame.init()
pygame.mixer.init()
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("扫雷游戏")
clock = pygame.time.Clock()
# 加载图像
tile_images = {
'hidden': pygame.image.load('images/tile_hidden.png').convert_alpha(),
'revealed': pygame.image.load('images/tile_revealed.png').convert_alpha(),
'bomb': pygame.image.load('images/tile_bomb.png').convert_alpha(),
'flag': pygame.image.load('images/tile_flag.png').convert_alpha()
}
for key, value in tile_images.items():
tile_images[key] = pygame.transform.scale(value, (TILESIZE, TILESIZE))
# 加载字体
font = pygame.font.Font(None, 24)
# 定义地图类
class Map:
def __init__(self, width, height, tilesize, bombs):
self.width = width
self.height = height
self.tilesize = tilesize
self.bombs = bombs
self.tiles = []
self.bomb_tiles = []
self.revealed_tiles = []
self.flagged_tiles = []
self.game_over = False
self.generate_tiles()
self.place_bombs()
self.update_tiles()
# 生成地图
def generate_tiles(self):
for x in range(self.width):
self.tiles.append([])
for y in range(self.height):
self.tiles[x].append(Tile(x, y, self.tilesize))
# 放置炸弹
def place_bombs(self):
for i in range(self.bombs):
while True:
x = random.randint(0, self.width - 1)
y = random.randint(0, self.height - 1)
if not self.tiles[x][y].is_bomb:
self.tiles[x][y].is_bomb = True
self.bomb_tiles.append((x, y))
break
# 更新所有方块的数字
def update_tiles(self):
for x in range(self.width):
for y in range(self.height):
count = 0
if not self.tiles[x][y].is_bomb:
for dx in range(-1, 2):
for dy in range(-1, 2):
if x + dx >= 0 and x + dx < self.width and y + dy >= 0 and y + dy < self.height:
if self.tiles[x + dx][y + dy].is_bomb:
count += 1
self.tiles[x][y].value = count
# 游戏结束
def gameover(self):
for x in range(self.width):
for y in range(self.height):
if self.tiles[x][y].is_bomb:
self.tiles[x][y].revealed = True
self.revealed_tiles.append((x, y))
self.game_over = True
# 标记方块
def flag_tile(self, x, y):
if not self.tiles[x][y].revealed:
if (x, y) in self.flagged_tiles:
self.flagged_tiles.remove((x, y))
else:
self.flagged_tiles.append((x, y))
# 翻开方块
def reveal_tile(self, x, y):
if not self.tiles[x][y].revealed:
self.tiles[x][y].revealed = True
self.revealed_tiles.append((x, y))
if self.tiles[x][y].is_bomb:
self.gameover()
elif self.tiles[x][y].value == 0:
for dx in range(-1, 2):
for dy in range(-1, 2):
if x + dx >= 0 and x + dx < self.width and y + dy >= 0 and y + dy < self.height:
if not self.tiles[x + dx][y + dy].is_bomb:
self.reveal_tile(x + dx, y + dy)
# 绘制地图
def draw(self, surface):
for x in range(self.width):
for y in range(self.height):
tile = self.tiles[x][y]
if tile.revealed:
surface.blit(tile_images['revealed'], (x * self.tilesize, y * self.tilesize))
if tile.is_bomb:
surface.blit(tile_images['bomb'], (x * self.tilesize, y * self.tilesize))
elif tile.value > 0:
text = font.render(str(tile.value), True, BLACK)
text_rect = text.get_rect()
text_rect.center = (x * self.tilesize + self.tilesize / 2, y * self.tilesize + self.tilesize / 2)
surface.blit(text, text_rect)
else:
surface.blit(tile_images['hidden'], (x * self.tilesize, y * self.tilesize))
if (x, y) in self.flagged_tiles:
surface.blit(tile_images['flag'], (x * self.tilesize, y * self.tilesize))
# 定义方块类
class Tile:
def __init__(self, x, y, size):
self.x = x
self.y = y
self.size = size
self.is_bomb = False
self.value = 0
self.revealed = False
# 判断鼠标是否在方块上
def is_clicked(self, mx, my):
return mx > self.x * self.size and mx < (self.x + 1) * self.size and my > self.y * self.size and my < (self.y + 1) * self.size
# 初始化地图
map = Map(WIDTH // TILESIZE, HEIGHT // TILESIZE, TILESIZE, BOMBS)
# 游戏主循环
running = True
while running:
# 事件处理
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.MOUSEBUTTONDOWN:
if event.button == 1:
for x in range(map.width):
for y in range(map.height):
if map.tiles[x][y].is_clicked(*event.pos):
map.reveal_tile(x, y)
elif event.button == 3:
for x in range(map.width):
for y in range(map.height):
if map.tiles[x][y].is_clicked(*event.pos):
map.flag_tile(x, y)
# 绘制背景
screen.fill(GREY)
# 绘制地图
map.draw(screen)
# 显示游戏结束信息
if map.game_over:
text = font.render("Game Over!", True, RED)
text_rect = text.get_rect()
text_rect.center = (WIDTH / 2, HEIGHT / 2)
screen.blit(text, text_rect)
# 更新屏幕
pygame.display.flip()
# 控制帧率
clock.tick(FPS)
# 退出游戏
pygame.quit()
```
在运行代码前,需要先准备好游戏所需的图片资源,并放置在名为 `images` 的文件夹中,然后将代码保存为 `minesweeper.py` 文件,执行命令 `python minesweeper.py` 即可开始游戏。
阅读全文
相关推荐
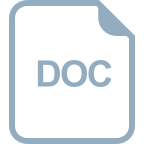
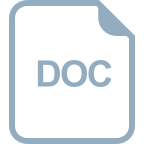
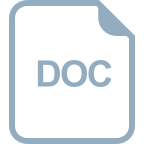



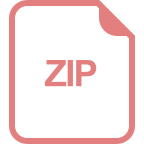
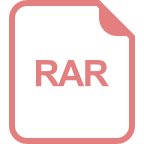
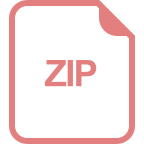







