用pygame做一个扫雷游戏
时间: 2023-08-19 17:50:03 浏览: 67
好的,下面是一个使用Pygame制作的简单扫雷游戏示例:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置游戏窗口的大小
screen_width = 400
screen_height = 400
screen = pygame.display.set_mode((screen_width, screen_height))
# 设置游戏标题
pygame.display.set_caption("扫雷游戏")
# 设置游戏背景颜色
background_color = (255, 255, 255)
# 设置游戏结束的标志
game_over = False
# 设置游戏时钟
clock = pygame.time.Clock()
# 定义方格的大小和数量
grid_size = 20
grid_count_x = screen_width // grid_size
grid_count_y = screen_height // grid_size
# 定义雷的数量
mine_count = 20
# 随机生成雷的位置
mines = []
for _ in range(mine_count):
mine = [random.randint(0, grid_count_x - 1), random.randint(0, grid_count_y - 1)]
if mine not in mines:
mines.append(mine)
# 定义方格状态
grid_status = [[0] * grid_count_x for _ in range(grid_count_y)] # 0代表未揭示,1代表揭示,2代表标记雷
# 游戏循环
while not game_over:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.MOUSEBUTTONDOWN:
if event.button == 1: # 左键点击
mouse_pos = pygame.mouse.get_pos()
grid_x = mouse_pos[0] // grid_size
grid_y = mouse_pos[1] // grid_size
if grid_status[grid_y][grid_x] == 0:
if [grid_x, grid_y] in mines:
# 点到雷,游戏结束
game_over = True
else:
# 统计周围雷的数量
count = 0
for dx in [-1, 0, 1]:
for dy in [-1, 0, 1]:
if dx == 0 and dy == 0:
continue
nx = grid_x + dx
ny = grid_y + dy
if nx >= 0 and nx < grid_count_x and ny >= 0 and ny < grid_count_y:
if [nx, ny] in mines:
count += 1
grid_status[grid_y][grid_x] = count + 1
elif event.button == 3: # 右键点击
mouse_pos = pygame.mouse.get_pos()
grid_x = mouse_pos[0] // grid_size
grid_y = mouse_pos[1] // grid_size
if grid_status[grid_y][grid_x] == 0:
grid_status[grid_y][grid_x] = 2
elif grid_status[grid_y][grid_x] == 2:
grid_status[grid_y][grid_x] = 0
# 绘制游戏界面
screen.fill(background_color)
for y in range(grid_count_y):
for x in range(grid_count_x):
rect = pygame.Rect(x * grid_size, y * grid_size, grid_size, grid_size)
pygame.draw.rect(screen, (192, 192, 192), rect)
if grid_status[y][x] == 1:
pygame.draw.rect(screen, (128, 128, 128), rect)
if [x, y] in mines:
pygame.draw.circle(screen, (0, 0, 0), (x * grid_size + grid_size // 2, y * grid_size + grid_size // 2), grid_size // 4)
else:
if grid_status[y][x] > 1:
font = pygame.font.SysFont(None, grid_size // 2)
text = font.render(str(grid_status[y][x] - 1), True, (0, 0, 0))
screen.blit(text, (x * grid_size + grid_size // 3, y * grid_size + grid_size // 3))
elif grid_status[y][x] == 2:
font = pygame.font.SysFont(None, grid_size // 2)
text = font.render("雷", True, (255, 0, 0))
screen.blit(text, (x * grid_size + grid_size // 3, y * grid_size + grid_size // 3))
# 更新游戏显示
pygame.display.update()
# 控制游戏帧率
clock.tick(30)
# 退出游戏
pygame.quit()
```
这个扫雷游戏使用方格来表示雷区,玩家可以通过左键点击方格来揭示方格,如果揭示到雷,游戏结束;如果揭示到的方格周围有雷,方格上方会显示雷的数量;玩家可以通过右键点击方格来标记可能的雷。游戏界面使用了灰色的方格表示未揭示的方格,揭示后的方格会变成浅灰色,雷会显示为黑色圆圈,标记雷的方格会显示红色的"雷"字样。
相关推荐
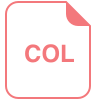
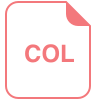
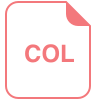
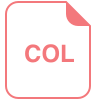
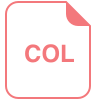









