用python写一个扫雷小游戏
时间: 2023-05-24 18:04:00 浏览: 126
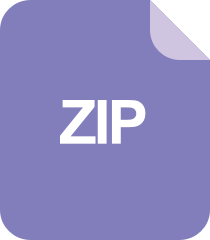
python编写的扫雷小游戏,包括源文件和打包好的可执行exe文件
好的,我可以帮你编写一个扫雷小游戏。首先,你需要安装`pygame`库来绘制游戏界面。可以通过以下命令来进行安装:
```
pip install pygame
```
接着,可以参考以下代码来编写扫雷小游戏:
```python
import pygame
import random
# 游戏界面的宽度和高度
WIDTH, HEIGHT = 600, 600
# 扫雷的行数和列数
ROWS, COLS = 10, 10
# 每个方块的宽度和高度
CELL_SIZE = 60
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GRAY = (128, 128, 128)
RED = (255, 0, 0)
# 初始化pygame
pygame.init()
# 创建游戏界面
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Minesweeper")
# 加载图像资源
mine_image = pygame.image.load("mine.png")
flag_image = pygame.image.load("flag.png")
# 创建方块类
class Cell:
def __init__(self, x, y):
self.x = x
self.y = y
self.is_mine = False
self.is_visible = False
self.is_flagged = False
self.mines_around = 0
def draw(self):
rect = pygame.Rect(self.x * CELL_SIZE, self.y * CELL_SIZE, CELL_SIZE, CELL_SIZE)
if self.is_visible:
pygame.draw.rect(screen, WHITE, rect)
if self.is_mine:
screen.blit(mine_image, rect)
else:
font = pygame.font.SysFont(None, 40)
text = font.render(str(self.mines_around), True, BLACK)
screen.blit(text, text.get_rect(center=rect.center))
else:
pygame.draw.rect(screen, GRAY, rect)
if self.is_flagged:
screen.blit(flag_image, rect)
def reveal(self):
self.is_visible = True
if self.is_mine:
return False
elif self.mines_around == 0:
for cell in get_neighbors(self.x, self.y):
if not cell.is_visible and cell.reveal() == False:
return False
return True
# 获取指定方块的八个邻居方块
def get_neighbors(x, y):
neighbors = []
for i in range(max(0, x - 1), min(ROWS, x + 2)):
for j in range(max(0, y - 1), min(COLS, y + 2)):
if i != x or j != y:
neighbors.append(cells[i][j])
return neighbors
# 随机生成指定数量的雷
def generate_mines(num_mines):
mines = set()
while len(mines) != num_mines:
x, y = random.randint(0, ROWS - 1), random.randint(0, COLS - 1)
if (x, y) not in mines:
mines.add((x, y))
cells[x][y].is_mine = True
# 更新每个方块周围雷的数量
def update_mines_around():
for i in range(ROWS):
for j in range(COLS):
if not cells[i][j].is_mine:
cells[i][j].mines_around = sum([1 for cell in get_neighbors(i, j) if cell.is_mine])
# 创建方块对象
cells = [[Cell(i, j) for j in range(COLS)] for i in range(ROWS)]
# 随机生成雷
generate_mines(10)
# 更新每个方块周围雷的数量
update_mines_around()
# 游戏主循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.MOUSEBUTTONUP:
if event.button == 1:
# 点击鼠标左键
x, y = event.pos[0] // CELL_SIZE, event.pos[1] // CELL_SIZE
if not cells[x][y].is_flagged:
if cells[x][y].reveal() == False:
# 游戏结束,显示所有雷
for row in cells:
for cell in row:
cell.is_visible = True
else:
# 判断游戏是否胜利
num_visible_cells = sum([1 for row in cells for cell in row if cell.is_visible])
num_mines = sum([1 for row in cells for cell in row if cell.is_mine])
if num_visible_cells == ROWS * COLS - num_mines:
# 游戏胜利,显示所有旗帜
for row in cells:
for cell in row:
if cell.is_mine:
cell.is_flagged = True
pygame.display.flip()
elif event.button == 3:
# 点击鼠标右键
x, y = event.pos[0] // CELL_SIZE, event.pos[1] // CELL_SIZE
if not cells[x][y].is_visible:
cells[x][y].is_flagged = not cells[x][y].is_flagged
pygame.display.flip()
# 绘制游戏界面
screen.fill(BLACK)
for row in cells:
for cell in row:
cell.draw()
pygame.display.flip()
# 退出pygame
pygame.quit()
```
在运行之前,你需要将`mine.png`和`flag.png`这两个图像文件放在与代码文件相同的目录下。这里提供两个图像文件作为示例,你也可以替换成你自己的图像。
如果一切正常,你应该能够看到如下的扫雷游戏界面:

阅读全文
相关推荐
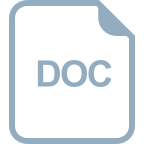
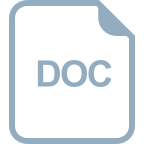















