用python写一个扫雷小游戏的代码
时间: 2023-12-29 11:19:21 浏览: 80
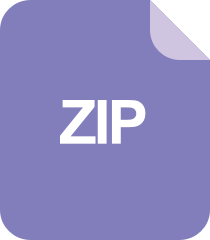
使用Python的扫雷游戏

以下是一个简单的扫雷游戏的 Python 代码,可以帮助您入门:
```python
import random
# 游戏板类
class Board:
def __init__(self, rows, cols, bombs):
self.rows = rows
self.cols = cols
self.bombs = bombs
self.board = [[" " for i in range(cols)] for j in range(rows)]
self.revealed_board = [[False for i in range(cols)] for j in range(rows)]
self.generate_board()
# 生成游戏板
def generate_board(self):
bombs_placed = 0
while bombs_placed < self.bombs:
row = random.randint(0, self.rows-1)
col = random.randint(0, self.cols-1)
if self.board[row][col] != "B":
self.board[row][col] = "B"
bombs_placed += 1
for row in range(self.rows):
for col in range(self.cols):
if self.board[row][col] != "B":
self.board[row][col] = str(self.get_adjacent_bombs(row, col))
# 获取相邻炸弹的数目
def get_adjacent_bombs(self, row, col):
count = 0
for i in range(-1, 2):
for j in range(-1, 2):
if i == 0 and j == 0:
continue
if row+i < 0 or row+i >= self.rows or col+j < 0 or col+j >= self.cols:
continue
if self.board[row+i][col+j] == "B":
count += 1
return count
# 打印游戏板
def print_board(self):
print(" ", end="")
for i in range(self.cols):
print(i, end=" ")
print()
for i in range(self.cols*2+2):
print("-", end="")
print()
for i in range(self.rows):
print(i, "|", end="")
for j in range(self.cols):
if self.revealed_board[i][j]:
print(self.board[i][j], end=" ")
else:
print(" ", end=" ")
print("|", end="")
print()
for j in range(self.cols*2+2):
print("-", end="")
print()
# 揭示游戏板
def reveal_board(self):
for i in range(self.rows):
for j in range(self.cols):
self.revealed_board[i][j] = True
# 检查是否获胜
def check_win(self):
for i in range(self.rows):
for j in range(self.cols):
if not self.revealed_board[i][j] and self.board[i][j] != "B":
return False
return True
# 游戏类
class Game:
def __init__(self, rows, cols, bombs):
self.board = Board(rows, cols, bombs)
self.game_over = False
# 运行游戏
def run(self):
while not self.game_over:
self.board.print_board()
self.get_move()
self.check_game_over()
self.board.reveal_board()
self.board.print_board()
if self.board.check_win():
print("你赢了!")
else:
print("你输了!")
# 获取玩家移动
def get_move(self):
valid_move = False
while not valid_move:
move = input("请输入行和列数(例如: 1 2): ").split()
if len(move) != 2:
print("输入无效!")
continue
row, col = move
if not row.isdigit() or not col.isdigit():
print("输入无效!")
continue
row, col = int(row), int(col)
if row < 0 or row >= self.board.rows or col < 0 or col >= self.board.cols:
print("输入无效!")
continue
if self.board.revealed_board[row][col]:
print("这个点已经被揭示了!")
continue
valid_move = True
self.make_move(row, col)
# 作出玩家移动
def make_move(self, row, col):
self.board.revealed_board[row][col] = True
if self.board.board[row][col] == "B":
self.game_over = True
# 检查游戏是否结束
def check_game_over(self):
if self.game_over:
return
for i in range(self.board.rows):
for j in range(self.board.cols):
if not self.board.revealed_board[i][j] and self.board.board[i][j] != "B":
return
self.game_over = True
# 运行游戏
game = Game(10, 10, 10)
game.run()
```
这是一个基本的扫雷游戏,您可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐















