帮我生成一个python的扫雷游戏
时间: 2023-07-25 13:52:34 浏览: 67
好的,这里提供一个简单的扫雷游戏的Python代码,你可以在此基础上进行修改和完善:
```python
import random
import pygame
# 初始化
pygame.init()
# 设置游戏窗口大小
screen = pygame.display.set_mode((240, 240))
# 设置游戏标题
pygame.display.set_caption("扫雷")
# 加载图片
tile = pygame.image.load("images/tile.png")
mine = pygame.image.load("images/mine.png")
flag = pygame.image.load("images/flag.png")
# 设置字体
font = pygame.font.Font(None, 24)
# 设置游戏变量
grid_size = 10
mine_count = 10
mine_list = []
tile_list = []
flag_list = []
game_over = False
game_win = False
# 随机生成地雷
for i in range(mine_count):
mine_x = random.randint(0, grid_size - 1)
mine_y = random.randint(0, grid_size - 1)
if (mine_x, mine_y) not in mine_list:
mine_list.append((mine_x, mine_y))
# 设置方格类
class Tile():
def __init__(self, x, y):
self.x = x
self.y = y
self.width = tile.get_rect().width
self.height = tile.get_rect().height
self.mine = False
self.adjacent_count = 0
self.revealed = False
self.flagged = False
def draw(self):
if self.flagged:
screen.blit(flag, (self.x * self.width, self.y * self.height))
elif not self.revealed:
screen.blit(tile, (self.x * self.width, self.y * self.height))
elif self.mine:
screen.blit(mine, (self.x * self.width, self.y * self.height))
else:
text = font.render(str(self.adjacent_count), True, (0, 0, 0))
screen.blit(text, (self.x * self.width + 5, self.y * self.height + 5))
# 初始化方格列表
for x in range(grid_size):
for y in range(grid_size):
tile_list.append(Tile(x, y))
# 计算每个方格周围地雷数量
for tile in tile_list:
if (tile.x, tile.y) in mine_list:
tile.mine = True
for i in range(-1, 2):
for j in range(-1, 2):
if (tile.x + i, tile.y + j) in mine_list:
tile.adjacent_count += 1
# 设置主循环
while True:
# 绘制方格
for tile in tile_list:
tile.draw()
# 判断游戏是否结束
if game_over:
text = font.render("Game Over", True, (255, 0, 0))
screen.blit(text, (80, 100))
elif game_win:
text = font.render("You Win", True, (0, 255, 0))
screen.blit(text, (80, 100))
# 事件处理
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.MOUSEBUTTONDOWN and not game_over and not game_win:
x, y = pygame.mouse.get_pos()
tile_x = x // tile.get_rect().width
tile_y = y // tile.get_rect().height
if event.button == 1:
if (tile_x, tile_y) in mine_list:
game_over = True
else:
tile_list[tile_y * grid_size + tile_x].revealed = True
if tile_list[tile_y * grid_size + tile_x].adjacent_count == 0:
for i in range(-1, 2):
for j in range(-1, 2):
if (tile_x + i, tile_y + j) not in mine_list and 0 <= tile_x + i < grid_size and 0 <= tile_y + j < grid_size:
tile_list[(tile_y + j) * grid_size + tile_x + i].revealed = True
elif event.button == 3:
flag_list.append((tile_x, tile_y))
tile_list[tile_y * grid_size + tile_x].flagged = True
# 绘制旗帜
for flag_x, flag_y in flag_list:
screen.blit(flag, (flag_x * tile.get_rect().width, flag_y * tile.get_rect().height))
# 判断是否胜利
if all(tile.revealed or tile.mine for tile in tile_list):
game_win = True
# 刷新屏幕
pygame.display.update()
```
你可以将这段代码保存为一个Python文件,然后将所需的图片放在`images`文件夹下运行。注意:本代码中没有设置计时器等功能,需要自己添加。
相关推荐
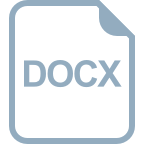
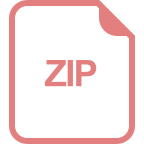
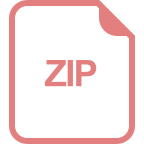














