python扫雷游戏代码_python实现扫雷游戏的示例
时间: 2023-07-13 19:32:37 浏览: 86
以下是一个简单的 Python 扫雷游戏代码示例:
```python
import random
# 定义常量
BOMB = -1
HIDDEN = 0
UNCOVERED = 1
class Minesweeper:
def __init__(self, rows, cols, bombs):
self.rows = rows
self.cols = cols
self.bombs = bombs
self.grid = [[HIDDEN] * cols for _ in range(rows)]
self.generate_bombs()
def generate_bombs(self):
bombs_placed = 0
while bombs_placed < self.bombs:
row = random.randint(0, self.rows - 1)
col = random.randint(0, self.cols - 1)
if self.grid[row][col] != BOMB:
self.grid[row][col] = BOMB
bombs_placed += 1
def uncover(self, row, col):
if self.grid[row][col] == BOMB:
return False
elif self.grid[row][col] == HIDDEN:
self.grid[row][col] = UNCOVERED
if self.neighbor_bomb_count(row, col) == 0:
for r in range(max(0, row - 1), min(row + 2, self.rows)):
for c in range(max(0, col - 1), min(col + 2, self.cols)):
if r != row or c != col:
self.uncover(r, c)
return True
def neighbor_bomb_count(self, row, col):
count = 0
for r in range(max(0, row - 1), min(row + 2, self.rows)):
for c in range(max(0, col - 1), min(col + 2, self.cols)):
if self.grid[r][c] == BOMB:
count += 1
return count
def __str__(self):
result = ''
for row in self.grid:
for cell in row:
if cell == UNCOVERED:
count = self.neighbor_bomb_count(self.grid.index(row), row.index(cell))
result += str(count) if count > 0 else ' '
elif cell == HIDDEN:
result += '*'
else:
result += 'X'
result += '\n'
return result[:-1]
# 示例用法
game = Minesweeper(5, 5, 5)
print(game)
game.uncover(2, 2)
print(game)
```
该示例代码使用了一个 `Minesweeper` 类来表示扫雷游戏,并包含了以下方法:
- `__init__(self, rows, cols, bombs)`:构造方法,初始化游戏的行数、列数和炸弹数,并生成游戏网格。
- `generate_bombs(self)`:随机生成指定数量的炸弹。
- `uncover(self, row, col)`:揭开指定位置的格子,并递归揭开周围的空格(如果有的话)。
- `neighbor_bomb_count(self, row, col)`:返回指定位置周围的炸弹数量。
- `__str__(self)`:将游戏网格转换为字符串,方便输出。
你可以根据自己的需求对这个示例代码进行修改和扩展。
相关推荐
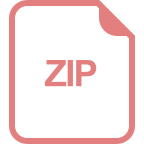
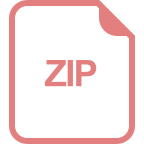













