写一个完整的扫雷游戏代码
时间: 2023-12-05 13:05:29 浏览: 41
好的,以下是一个完整的扫雷游戏代码,包括计算周围雷数、判断游戏胜利条件、点击雷时的游戏失败等功能:
```python
import pygame
import random
# 游戏窗口大小
WINDOW_WIDTH, WINDOW_HEIGHT = 400, 400
# 方块大小和行列数
BLOCK_SIZE = 20
ROWS, COLS = WINDOW_HEIGHT // BLOCK_SIZE, WINDOW_WIDTH // BLOCK_SIZE
# 雷的数量
NUM_MINES = 40
# 初始化Pygame
pygame.init()
# 创建游戏窗口
window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
# 设置窗口标题
pygame.display.set_caption("扫雷游戏")
# 定义颜色
BLACK = (0, 0, 0)
GRAY = (128, 128, 128)
WHITE = (255, 255, 255)
# 游戏结束标志
game_over = False
# 创建方块类
class Block:
def __init__(self, row, col):
self.row = row
self.col = col
self.x = col * BLOCK_SIZE
self.y = row * BLOCK_SIZE
self.is_mine = False
self.is_revealed = False
self.is_flagged = False
self.adjacent_mines = 0
def draw(self):
if self.is_revealed:
pygame.draw.rect(window, GRAY, (self.x, self.y, BLOCK_SIZE, BLOCK_SIZE))
if self.is_mine:
pygame.draw.circle(window, BLACK, (self.x + BLOCK_SIZE // 2, self.y + BLOCK_SIZE // 2), BLOCK_SIZE // 4)
elif self.adjacent_mines > 0:
font = pygame.font.Font(None, BLOCK_SIZE)
text = font.render(str(self.adjacent_mines), True, BLACK)
text_rect = text.get_rect(center=(self.x + BLOCK_SIZE // 2, self.y + BLOCK_SIZE // 2))
window.blit(text, text_rect)
else:
pygame.draw.rect(window, WHITE, (self.x, self.y, BLOCK_SIZE, BLOCK_SIZE))
pygame.draw.rect(window, GRAY, (self.x, self.y, BLOCK_SIZE, BLOCK_SIZE), 1)
if self.is_flagged:
pygame.draw.line(window, BLACK, (self.x + BLOCK_SIZE // 2, self.y + BLOCK_SIZE // 4),
(self.x + BLOCK_SIZE // 2, self.y + BLOCK_SIZE * 3 // 4), 2)
pygame.draw.line(window, BLACK, (self.x + BLOCK_SIZE // 4, self.y + BLOCK_SIZE // 2),
(self.x + BLOCK_SIZE * 3 // 4, self.y + BLOCK_SIZE // 2), 2)
def reveal(self):
self.is_revealed = True
# 创建方块列表
blocks = [[Block(row, col) for col in range(COLS)] for row in range(ROWS)]
# 随机生成雷的位置
mines = random.sample(range(ROWS * COLS), NUM_MINES)
for mine in mines:
row = mine // COLS
col = mine % COLS
blocks[row][col].is_mine = True
# 计算每个方块周围的雷数
for row in range(ROWS):
for col in range(COLS):
block = blocks[row][col]
if not block.is_mine:
count = 0
for i in range(max(0, row - 1), min(row + 2, ROWS)):
for j in range(max(0, col - 1), min(col + 2, COLS)):
if blocks[i][j].is_mine:
count += 1
block.adjacent_mines = count
# 游戏主循环
while not game_over:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if event.type == pygame.MOUSEBUTTONDOWN:
x, y = pygame.mouse.get_pos()
row = y // BLOCK_SIZE
col = x // BLOCK_SIZE
if pygame.mouse.get_pressed()[0]: # 左键点击
if not blocks[row][col].is_flagged:
blocks[row][col].reveal()
if blocks[row][col].is_mine:
game_over = True
elif pygame.mouse.get_pressed()[2]: # 右键点击
blocks[row][col].is_flagged = not blocks[row][col].is_flagged
# 判断游戏胜利条件
def check_win():
for row in blocks:
for block in row:
if not block.is_revealed and not block.is_mine:
return False
return True
# 绘制方块
for row in blocks:
for block in row:
block.draw()
# 判断游戏胜利或失败
if game_over:
font = pygame.font.Font(None, 50)
if check_win():
text = font.render("You Win!", True, BLACK)
else:
text = font.render("Game Over!", True, BLACK)
text_rect = text.get_rect(center=(WINDOW_WIDTH // 2, WINDOW_HEIGHT // 2))
window.blit(text, text_rect)
# 更新屏幕
pygame.display.update()
# 退出游戏
pygame.quit()
```
这个代码实现了完整的扫雷游戏,包括生成随机雷的位置、计算周围雷数、左键点击方块进行揭示和右键点击进行标记等功能。当游戏结束时,会显示相应的胜利或失败信息。你可以尝试运行代码并进行游戏。希望对你有帮助!
相关推荐
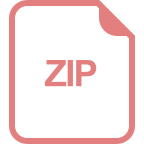
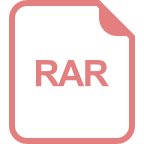













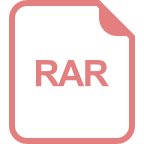