堆栈(Stack)是一种特殊的顺序存储表,只允许在表的一端(栈顶)进行插入和删除操作,另一端(栈底)则不允许插入和删除操作,堆栈的插入和删除通常称为入栈和出栈。栈顶的当前位置是动态的,用一个指针,即栈顶指针来标示栈顶当前位置。如下图所示: 堆栈可以用数组来表示,假设堆栈可保存的数据类型为char。那么堆栈数据成员如下: char element[MAXSIZE]; int index; //堆栈指针 堆栈相关的成员函数如下: void push(char item); //元素item进栈,即插入item到栈顶; void pop( ); //弹出栈顶元素; char top(); //返回栈顶元素的值,但不移动栈顶指针; bool isEmpty(); //判断堆栈是否为空 解决问题: (1)根据上述提示实现一个堆栈;注意栈空和栈满情况的处理; (2)堆栈在计算机语言中的编译过程中用来进行语法检查,编写一个程序,利用堆栈来检查一个C/C++语言程序中的花括号、方括号和圆括号是否配对。
时间: 2023-12-03 20:45:39 浏览: 78
(1) 堆栈的实现:
```C++
#include <iostream>
using namespace std;
const int MAXSIZE = 100; // 堆栈的最大容量
class Stack {
private:
char element[MAXSIZE];
int index; // 栈顶指针
public:
Stack() { index = -1; } // 构造函数,初始化栈顶指针为-1
void push(char item); // 元素item进栈,即插入item到栈顶
void pop(); // 弹出栈顶元素
char top(); // 返回栈顶元素的值,但不移动栈顶指针
bool isEmpty(); // 判断堆栈是否为空
};
void Stack::push(char item) {
if (index >= MAXSIZE - 1) {
cout << "Stack is full!" << endl;
return;
}
index++;
element[index] = item;
}
void Stack::pop() {
if (index < 0) {
cout << "Stack is empty!" << endl;
return;
}
index--;
}
char Stack::top() {
if (index < 0) {
cout << "Stack is empty!" << endl;
return '\0';
}
return element[index];
}
bool Stack::isEmpty() {
return (index == -1);
}
int main() {
Stack s;
s.push('a');
s.push('b');
s.push('c');
cout << s.top() << endl; // 输出c
s.pop();
cout << s.top() << endl; // 输出b
s.pop();
cout << s.top() << endl; // 输出a
s.pop();
cout << s.top() << endl; // 输出Stack is empty!,并且返回'\0',表示栈已经为空
return 0;
}
```
(2) 利用堆栈来检查一个C/C++语言程序中的花括号、方括号和圆括号是否配对:
```C++
#include <iostream>
#include <stack>
#include <string>
using namespace std;
bool isMatching(char left, char right) {
if (left == '(' && right == ')') return true;
if (left == '[' && right == ']') return true;
if (left == '{' && right == '}') return true;
return false;
}
bool isValid(string s) {
stack<char> st;
for (int i = 0; i < s.length(); i++) {
if (s[i] == '(' || s[i] == '[' || s[i] == '{') {
st.push(s[i]);
} else if (s[i] == ')' || s[i] == ']' || s[i] == '}') {
if (st.empty() || !isMatching(st.top(), s[i])) {
return false;
}
st.pop();
}
}
return st.empty();
}
int main() {
string s = "{[(])}";
if (isValid(s)) {
cout << "Valid!" << endl;
} else {
cout << "Invalid!" << endl;
}
return 0;
}
```
例子中利用了STL中的stack容器来实现堆栈。在遍历字符串时,如果遇到左括号,就将其压入堆栈中;如果遇到右括号,就判断堆栈是否为空,如果为空或者栈顶的左括号与当前的右括号不匹配,则说明括号不配对,返回false。最后,如果堆栈为空,则说明所有括号都配对,返回true。
阅读全文
相关推荐
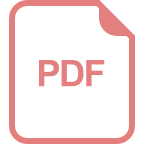
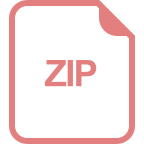
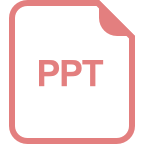















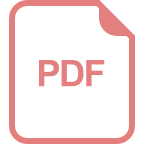