将这个代码改为C语言,使其能再codeblocks里能运行
时间: 2024-09-13 21:06:47 浏览: 45
将上述Python代码转换为C语言并在CodeBlocks中运行,需要使用`stdio.h`, `stdlib.h` 和 `string.h`头文件,并创建一个结构体来表示学生信息。下面是一个简化的C语言版本:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct {
char id[10];
char name[20];
int scores[3]; // 课程成绩数组
int total_score;
} Student;
void add_student(Student* students, int n) {
Student new_student;
scanf("%s %s", new_student.id, new_student.name);
for (int i = 0; i < 3; i++) {
scanf("%d", &new_student.scores[i]);
}
new_student.total_score = 0;
for (int i = 0; i < 3; i++) {
new_student.total_score += new_student.scores[i];
}
students[n] = new_student;
}
double calculate_average(const Student* students, int n) {
double total_avg = 0.0;
for (int i = 0; i < n; i++) {
total_avg += students[i].total_score;
}
return total_avg / n;
}
void find_max_student(Student* students, int n, Student* max_student) {
*max_student = students[0];
for (int i = 1; i < n; i++) {
if (students[i].total_score > max_student->total_score) {
*max_student = students[i];
}
}
}
int main() {
int n, i;
printf("请输入学生数量: ");
scanf("%d", &n);
Student* students = malloc(n * sizeof(Student));
add_student(students, n); // 添加学生
double avg_scores[] = calculate_average(students, n);
printf("各门课的平均成绩: ");
for (i = 0; i < 3; i++) {
printf("%.0f ", avg_scores[i]);
}
Student max_student;
find_max_student(students, n, &max_student);
printf("\n最高分学生信息: %s %s %d %d %d\n",
max_student.id, max_student.name, max_student.scores[0], max_student.scores[1], max_student.scores[2]);
free(students);
return 0;
}
```
注意:在CodeBlocks中编译和运行此C代码,你需要确保已经安装了`gcc`编译器,并配置了正确的路径,以便可以链接和运行生成的二进制文件。记得保存代码到`.c`文件,然后在CodeBlocks的构建菜单中选择合适的选项进行编译和运行。
阅读全文
相关推荐
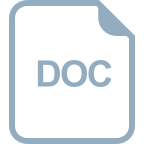
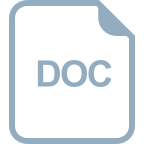
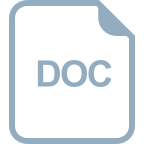
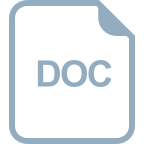
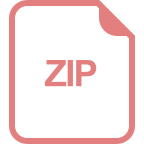
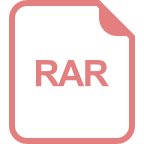
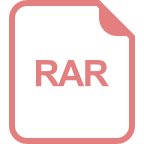
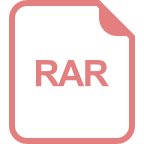
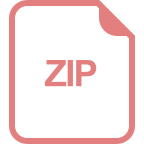
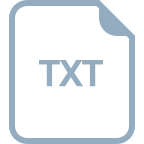
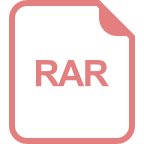
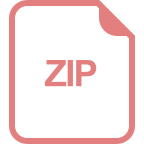
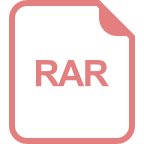
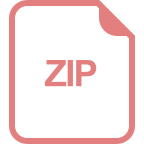
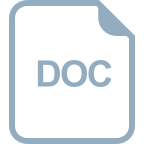
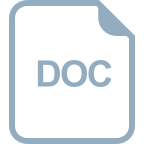


