c语言模拟实现shell命令
时间: 2023-05-01 16:02:44 浏览: 82
b'c\xe8\xaf\xad\xe8\xa8\x80\xe6\xa8\xa1\xe6\x8b\x9f\xe5\xae\x9e\xe7\x8e\xb0shell\xe5\x91\xbd\xe4\xbb\xa4' 的意思是 "用C语言模拟实现shell命令"。
相关问题
linux用C语言模拟shell命令并且实现重定向
要在 Linux 上使用 C 语言模拟 shell 命令,可以使用系统调用函数来执行命令。其中,`fork()` 函数用于创建子进程,`execvp()` 函数用于在子进程中执行命令。以下是一个简单的示例:
```c
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <string.h>
#include <sys/wait.h>
#include <fcntl.h>
#define MAX_ARGS 10
#define MAX_COMMAND_LENGTH 50
int main() {
char command[MAX_COMMAND_LENGTH];
char *args[MAX_ARGS];
int background = 0;
int redirect = 0;
char *redirect_file;
while (1) {
// 读取用户输入的命令
printf("myshell> ");
fgets(command, MAX_COMMAND_LENGTH, stdin);
command[strlen(command)-1] = '\0'; // 去掉换行符
// 解析命令参数
int i = 0;
args[i] = strtok(command, " ");
while (args[i] != NULL) {
i++;
args[i] = strtok(NULL, " ");
}
args[i] = NULL;
// 判断是否后台运行
if (args[i-1] != NULL && strcmp(args[i-1], "&") == 0) {
background = 1;
args[i-1] = NULL;
}
// 判断是否重定向输出
for (int j = 0; j < i; j++) {
if (strcmp(args[j], ">") == 0) {
redirect = 1;
args[j] = NULL;
redirect_file = args[j+1];
}
}
// 创建子进程执行命令
pid_t pid = fork();
if (pid == -1) {
perror("fork error");
exit(1);
} else if (pid == 0) {
// 子进程
if (redirect) {
int fd = open(redirect_file, O_WRONLY | O_CREAT | O_TRUNC, 0666);
if (fd == -1) {
perror("open error");
exit(1);
}
dup2(fd, STDOUT_FILENO);
close(fd);
}
execvp(args[0], args);
perror("execvp error");
exit(1);
} else {
// 父进程
if (!background) {
waitpid(pid, NULL, 0);
}
}
}
return 0;
}
```
上述代码实现了一个简单的 shell,支持后台运行和重定向输出。例如,可以输入 `ls -l > output.txt` 命令将 `ls -l` 命令的输出重定向到 `output.txt` 文件中。
linux用C语言模拟shell命令实现<>重定向功能
在 Linux 中,我们可以使用 C 语言中的系统调用和文件操作函数来模拟 Shell 命令的重定向功能。其中 `<` 符号表示从文件中读取输入,而 `>` 符号表示将输出写入文件。
具体实现步骤如下:
1. 使用 `open()` 函数打开文件并获得文件描述符,`open()` 函数的第一个参数是要打开的文件名,第二个参数是文件打开的模式,比如 `O_RDONLY` 表示只读模式,`O_CREAT` 表示如果文件不存在就创建它。
```c
int fd = open("input.txt", O_RDONLY);
```
2. 使用 `dup2()` 函数将文件描述符重定向到标准输入或标准输出,`dup2()` 函数的第一个参数是要重定向的文件描述符,第二个参数是要重定向到的文件描述符,例如将标准输入重定向到文件描述符 `fd`:
```c
dup2(fd, 0); // 将标准输入重定向到文件描述符fd
```
3. 使用 `close()` 函数关闭文件描述符:
```c
close(fd); // 关闭文件描述符
```
完整的代码实现如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
int fd;
char buf[1024];
// 从文件中读取输入
fd = open("input.txt", O_RDONLY);
dup2(fd, 0); // 将标准输入重定向到文件描述符fd
close(fd); // 关闭文件描述符
// 输出到文件中
fd = open("output.txt", O_WRONLY | O_CREAT | O_TRUNC, 0666);
dup2(fd, 1); // 将标准输出重定向到文件描述符fd
close(fd); // 关闭文件描述符
// 读取标准输入并写入标准输出
while (fgets(buf, sizeof(buf), stdin)) {
printf("%s", buf);
}
return 0;
}
```
在命令行中运行该程序,可以将输入文件的内容输出到标准输出,并将标准输出写入输出文件中:
```
./redirect < input.txt > output.txt
```
相关推荐
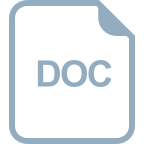
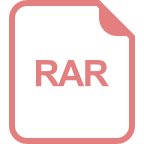












