import processing.serial.*; Serial myPort; PImage ocean = new PImage(); void setup() { myPort = new Serial(this, "COM3", 9600); size(1280, 720); ocean = loadImage("ocean.jpg"); ocean.resize(width, height); } void draw() { image(ocean, 0, 0); loadPixels(); ocean.loadPixels(); while (myPort.available() > 0) { char inByte = myPort.readChar(); println(inByte); switch (inByte) { case '3': for (int x = 0; x < width - 1; x++) { for (int y = 0; y < height - 1; y++) { int loc1 = x + y * width; int loc2 = (x + 1) + y * width; float b1 = brightness(ocean.pixels[loc1]); float b2 = brightness(ocean.pixels[loc2]); float diff = abs(b1 - b2); if (diff > 15) { pixels[loc1] = color(0); } else { pixels[loc1] = color(255); } } } updatePixels(); break; default: // 如果没有匹配到任何条件,则执行该语句块 break; } } }解释这段代码
时间: 2024-01-23 14:02:32 浏览: 82
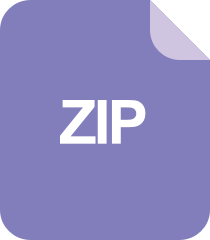
c++跨平台串口库serial
这段代码使用 Processing 编写,主要功能是读取串口数据并显示一张图片,当接收到字符 '3' 时,会对图片进行二值化处理。
具体解释如下:
1. 导入所需的库
```
import processing.serial.*; // 用于串口通信
```
2. 声明变量
```
Serial myPort; // 串口对象
PImage ocean = new PImage(); // 图片对象
```
3. 设置窗口大小并加载图片
```
void setup() {
myPort = new Serial(this, "COM3", 9600); // 初始化串口对象
size(1280, 720); // 设置窗口大小
ocean = loadImage("ocean.jpg"); // 加载图片
ocean.resize(width, height); // 调整图片大小以适应窗口
}
```
4. 在窗口中显示图片
```
void draw() {
image(ocean, 0, 0); // 在窗口中显示图片
}
```
5. 读取串口数据并进行二值化处理
```
while (myPort.available() > 0) { // 如果有可用的串口数据
char inByte = myPort.readChar(); // 读取一个字符
switch (inByte) { // 判断字符
case '3': // 如果字符为 '3'
for (int x = 0; x < width - 1; x++) { // 遍历图片像素
for (int y = 0; y < height - 1; y++) {
int loc1 = x + y * width; // 计算像素位置
int loc2 = (x + 1) + y * width;
float b1 = brightness(ocean.pixels[loc1]); // 获取像素亮度值
float b2 = brightness(ocean.pixels[loc2]);
float diff = abs(b1 - b2); // 计算相邻像素亮度差
if (diff > 15) { // 如果亮度差大于阈值
pixels[loc1] = color(0); // 将像素设为黑色
} else {
pixels[loc1] = color(255); // 否则将像素设为白色
}
}
}
updatePixels(); // 更新像素数组
break;
default: // 如果字符不为 '3',则不做任何处理
break;
}
}
```
通过以上代码,可以实现读取串口数据并在窗口中显示图片,当接收到字符 '3' 时,会对图片进行简单的二值化处理。当然,这只是一个示例,您可以根据具体需求进行更改和扩展。
阅读全文
相关推荐
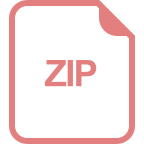
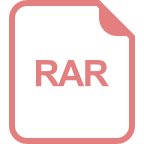

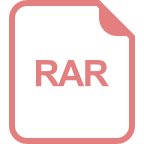
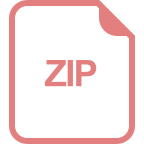
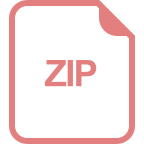
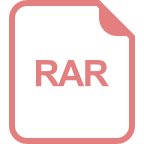










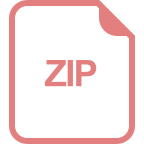