import processing.serial.*; Serial myPort; PImage ocean = new PImage(); void setup() { myPort = new Serial(this, "COM3", 9600); size(1280,720); ocean = loadImage("ocean.jpg"); ocean.resize(width, height); } void draw() { image(ocean, 0, 0); loadPixels(); ocean.loadPixels(); while (myPort.available() > 0) { char inByte = myPort.readChar(); println(inByte); switch(inByte){ case'1': for (int x=0; x<width-1; x++) { for (int y=0; y<height; y++) { int loc1 = x+y*width; int loc2 = (x+1)+y*width; float b1 = brightness(ocean.pixels[loc1]); float b2 = brightness(ocean.pixels[loc2]); float diff = abs(b1-b2); if (diff>15) { pixels[loc1] =color(0); } else { pixels[loc1] =color(255); } } updatePixels(); } break; } } }这段代码有什么问题
时间: 2024-01-23 15:04:09 浏览: 22
这段代码看起来没有明显的语法错误,但是有一些问题需要注意:
1. 在 `for` 循环中,y 的循环次数应该是 `height-1`,否则会出现数组越界的问题;
2. 在 `for` 循环中,x 的循环次数应该是 `width-1`,否则会出现数组越界的问题;
3. 在 `for` 循环中,应该先对 `ocean` 图像进行像素值的比较,再根据比较结果修改 `pixels` 数组中对应像素的颜色值;
4. 在 `case` 语句中,如果没有匹配到任何条件,应该添加一个 `default` 分支,以避免出现未知错误。
下面是修改后的代码:
```java
import processing.serial.*;
Serial myPort;
PImage ocean = new PImage();
void setup() {
myPort = new Serial(this, "COM3", 9600);
size(1280, 720);
ocean = loadImage("ocean.jpg");
ocean.resize(width, height);
}
void draw() {
image(ocean, 0, 0);
loadPixels();
ocean.loadPixels();
while (myPort.available() > 0) {
char inByte = myPort.readChar();
println(inByte);
switch (inByte) {
case '1':
for (int x = 0; x < width - 1; x++) {
for (int y = 0; y < height - 1; y++) {
int loc1 = x + y * width;
int loc2 = (x + 1) + y * width;
float b1 = brightness(ocean.pixels[loc1]);
float b2 = brightness(ocean.pixels[loc2]);
float diff = abs(b1 - b2);
if (diff > 15) {
pixels[loc1] = color(0);
} else {
pixels[loc1] = color(255);
}
}
}
updatePixels();
break;
default:
// 如果没有匹配到任何条件,则执行该语句块
break;
}
}
}
```
请注意,这只是一个简单的示例代码,具体实现方式可能需要根据你的项目需求进行修改。
相关推荐
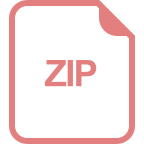
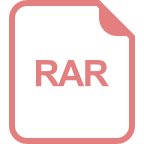














