python计算二值化图像中封闭区域面积
时间: 2024-09-28 19:07:05 浏览: 25
在Python中,处理二值化图像并计算封闭区域面积通常会涉及图像处理库如PIL(Python Imaging Library)或OpenCV。以下是一个基本步骤:
1. **读取图像**:首先使用PIL读取图片文件。
```python
from PIL import Image
img = Image.open('binary_image.png')
```
2. **二值化处理**:将图像转换为黑白二值图像,常见的方法有阈值分割、Otsu's方法等。
```python
# 使用Otsu's方法二值化
from skimage.filters import threshold_otsu
threshold = threshold_otsu(img)
binary_img = img > threshold
```
3. **膨胀操作**:为了消除小的噪声点,并连接一些孤立的像素,可以对二值图像进行膨胀操作。
```python
import numpy as np
from scipy.ndimage.morphology import binary_dilation
binary_img = binary_dilation(binary_img)
```
4. **找到轮廓**:通过轮廓检测找出图像的边界。
```python
from skimage.measure import find_contours
contours = find_contours(binary_img, 0.5) # 假设0.5是近似轮廓阈值
```
5. **计算面积**:对于每个轮廓,可以用`numpy`或`scipy`库计算其面积。
```python
areas = [np.sum(c[:, 0] * c[:, 1]) for c in contours]
total_area = sum(areas)
```
6. **显示结果**:如果需要,可以绘制原始图像和轮廓,以及总面积。
```python
import matplotlib.pyplot as plt
plt.imshow(binary_img, cmap='gray')
for contour in contours:
plt.plot(contour[:, 1], contour[:, 0], linewidth=2)
plt.show()
```
相关推荐
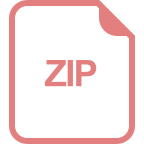
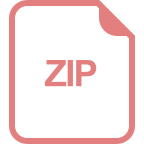
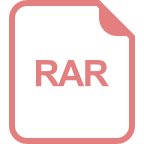















