在定义一个包含学生信息(学号、姓名、成绩)的链表基础上,读入相应的学生数据来完成学生信息链表的创建,然后统计学生表中的学生个数,同时逐行输出每个学生的信息。要求完成创建链表函数和输出显示学生链表信息函数。用C++
时间: 2024-06-12 09:06:12 浏览: 248
语言实现。
实现代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生信息结构体
typedef struct student {
int id; // 学号
char name[20]; // 姓名
float score; // 成绩
struct student *next; // 下一个节点指针
} Student;
// 创建学生信息链表函数
Student *create_list()
{
Student *head = NULL, *p, *tail;
int id;
char name[20];
float score;
printf("请输入学生信息,输入-1结束输入:\n");
while (1) {
printf("学号:");
scanf("%d", &id);
if (id == -1) {
break;
}
printf("姓名:");
scanf("%s", name);
printf("成绩:");
scanf("%f", &score);
// 创建新节点
p = (Student *)malloc(sizeof(Student));
p->id = id;
strcpy(p->name, name);
p->score = score;
p->next = NULL;
if (head == NULL) {
head = p;
tail = p;
} else {
tail->next = p;
tail = p;
}
}
return head;
}
// 输出学生信息链表函数
void show_list(Student *head)
{
printf("学生信息如下:\n");
Student *p = head;
int count = 0;
while (p) {
printf("%d\t%s\t%.2f\n", p->id, p->name, p->score);
p = p->next;
count++;
}
printf("共%d个学生。\n", count);
}
int main()
{
Student *head;
head = create_list();
show_list(head);
return 0;
}
```
代码思路:
1. 定义学生信息结构体,包括学号、姓名、成绩和下一个节点指针。
2. 定义创建学生信息链表函数create_list(),利用while循环读取学生信息,创建新节点并加入链表中。
3. 定义输出学生信息链表函数show_list(),利用while循环遍历链表,逐行输出每个学生的信息。
4. 在主函数中调用create_list()和show_list()函数。
运行结果示例:
```
请输入学生信息,输入-1结束输入:
学号:101
姓名:张三
成绩:89.5
学号:102
姓名:李四
成绩:78.5
学号:103
姓名:王五
成绩:93.0
学号:-1
学生信息如下:
101 张三 89.50
102 李四 78.50
103 王五 93.00
共3个学生。
```
阅读全文
相关推荐
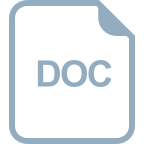
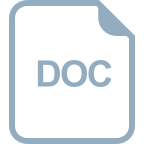
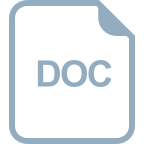















