用C++构建一个班级信息链表类,学生信息包括:学号(ID)、姓名(Name)、出生 年月(Birth)、性别(Sex)和某门功课成绩(Score)。类的成员函数只需要满足本 题要求即可,学生人数由用户决定。为避免大量输入工作,建议学生信息从文件 读入,文件格式可自行设定并描述清楚。实现:(1)按成绩降序排列,(2)汇总 各分数段人数。分数段划分区间为[90,100],[80,90),[70,80), [60,70), [0,60),(3) 列出最高分和最低分的同学信息。(注:不允许使用 STL)
时间: 2024-03-08 10:48:08 浏览: 85
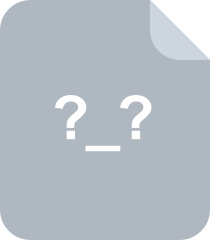
C++ 链表 学生类 班级类

以下是用C++构建一个班级信息链表类的代码:
```c++
#include <iostream>
#include <fstream>
#include <cstring>
using namespace std;
// 学生信息结构体
struct Student{
char ID[20]; //学号
char Name[20]; //姓名
char Birth[20]; //出生年月
char Sex[10]; //性别
float Score; //某门功课成绩
Student* next; //指向下一个学生的指针
};
class StudentList{
private:
Student* head; //链表头指针
public:
StudentList(){ head = NULL; } //构造函数
~StudentList(); //析构函数
void ReadDataFromFile(const char* filename); //从文件读取学生信息
void AddStudent(Student* p); //添加一个学生
void SortByScore(); //按成绩降序排列
void PrintScoreSummary(); //汇总各分数段人数
void PrintMaxMinScore(); //列出最高分和最低分的同学信息
};
StudentList::~StudentList(){
Student* p = head;
while(p){
Student* q = p->next;
delete p;
p = q;
}
}
void StudentList::ReadDataFromFile(const char* filename){
ifstream infile(filename);
if(!infile){
cout << "Failed to open file " << filename << endl;
return;
}
char line[100];
while(infile.getline(line, 100)){
char* token;
Student* p = new Student;
token = strtok(line, ",");
strcpy(p->ID, token);
token = strtok(NULL, ",");
strcpy(p->Name, token);
token = strtok(NULL, ",");
strcpy(p->Birth, token);
token = strtok(NULL, ",");
strcpy(p->Sex, token);
token = strtok(NULL, ",");
p->Score = atof(token);
AddStudent(p);
}
infile.close();
}
void StudentList::AddStudent(Student* p){
if(!head){
head = p;
p->next = NULL;
}
else{
Student* q = head;
while(q->next) q = q->next;
q->next = p;
p->next = NULL;
}
}
void StudentList::SortByScore(){
Student* p = head;
while(p){
Student* q = p->next;
while(q){
if(p->Score < q->Score){
//交换学生信息
Student tmp;
memcpy(&tmp, p, sizeof(Student));
memcpy(p, q, sizeof(Student));
memcpy(q, &tmp, sizeof(Student));
}
q = q->next;
}
p = p->next;
}
}
void StudentList::PrintScoreSummary(){
int count[6] = {0}; //分数段人数计数器
Student* p = head;
while(p){
if(p->Score >= 90) count[0]++;
else if(p->Score >= 80) count[1]++;
else if(p->Score >= 70) count[2]++;
else if(p->Score >= 60) count[3]++;
else count[4]++;
p = p->next;
}
count[5] = count[4]; //最后一个分数段人数为[0,60]
cout << "Score summary:" << endl;
cout << "[90,100]: " << count[0] << endl;
cout << "[80,90): " << count[1] << endl;
cout << "[70,80): " << count[2] << endl;
cout << "[60,70): " << count[3] << endl;
cout << "[0,60): " << count[4] << endl;
}
void StudentList::PrintMaxMinScore(){
Student* maxp = head;
Student* minp = head;
Student* p = head;
while(p){
if(p->Score > maxp->Score) maxp = p;
if(p->Score < minp->Score) minp = p;
p = p->next;
}
cout << "Max score student: " << maxp->ID << "\t" << maxp->Name << "\t" << maxp->Birth << "\t" << maxp->Sex << "\t" << maxp->Score << endl;
cout << "Min score student: " << minp->ID << "\t" << minp->Name << "\t" << minp->Birth << "\t" << minp->Sex << "\t" << minp->Score << endl;
}
int main(){
StudentList list;
list.ReadDataFromFile("students.txt"); //从文件读取学生信息
list.SortByScore(); //按成绩降序排列
list.PrintScoreSummary(); //汇总各分数段人数
list.PrintMaxMinScore(); //列出最高分和最低分的同学信息
return 0;
}
```
在上述代码中,我们首先定义了一个学生信息结构体Student,其中包括了学号、姓名、出生年月、性别和某门功课成绩等信息。然后我们定义了一个班级信息链表类StudentList,其中包括了读取学生信息、添加学生、按成绩降序排列、汇总各分数段人数和列出最高分和最低分的同学信息等成员函数。
在main函数中,我们创建了一个StudentList对象list,并通过ReadDataFromFile函数从文件中读取学生信息。然后我们按成绩降序排列、汇总各分数段人数和列出最高分和最低分的同学信息,最后程序结束。
需要注意的是,在我们的实现中,我们没有使用STL,而是手动实现了链表操作。
阅读全文
相关推荐









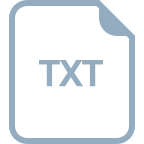
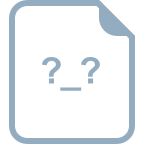
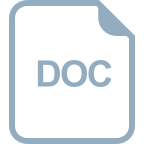
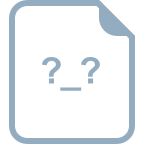
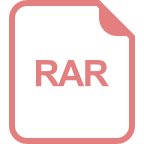