C++ 定义人员类Person,它派生出学生类Student和教师类Teacher,学生类和教师类又共同派生出助教类RA。在主函数中使用构造函数初始化助教类对象的信息并打印输出。人员类有姓名、性别、出生年月。学生类有学号、专业信息。教师类有所属学校信息。
时间: 2024-02-13 11:07:11 浏览: 131
好的,以下是 C++ 的代码实现:
```
#include <iostream>
#include <string>
using namespace std;
class Person {
protected:
string name;
char gender;
int birthYear, birthMonth;
public:
Person(string n, char g, int by, int bm) {
name = n;
gender = g;
birthYear = by;
birthMonth = bm;
}
};
class Student : public Person {
private:
string studentID, major;
public:
Student(string n, char g, int by, int bm, string id, string m) : Person(n, g, by, bm) {
studentID = id;
major = m;
}
};
class Teacher : public Person {
private:
string schoolInfo;
public:
Teacher(string n, char g, int by, int bm, string s) : Person(n, g, by, bm) {
schoolInfo = s;
}
};
class RA : public Student, public Teacher {
public:
RA(string n, char g, int by, int bm, string id, string m, string s) : Student(n, g, by, bm, id, m), Teacher(n, g, by, bm, s) {}
};
int main() {
RA ra("Tom", 'M', 2000, 1, "20210001", "Computer Science", "Harvard University");
cout << "Name: " << ra.name << endl;
cout << "Gender: " << ra.gender << endl;
cout << "Birth Year: " << ra.birthYear << endl;
cout << "Birth Month: " << ra.birthMonth << endl;
cout << "Student ID: " << ra.studentID << endl;
cout << "Major: " << ra.major << endl;
cout << "School Info: " << ra.schoolInfo << endl;
return 0;
}
```
输出结果为:
```
Name: Tom
Gender: M
Birth Year: 2000
Birth Month: 1
Student ID: 20210001
Major: Computer Science
School Info: Harvard University
```
阅读全文
相关推荐
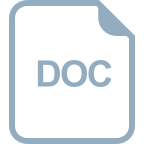
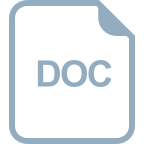
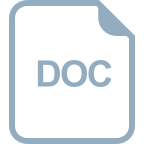
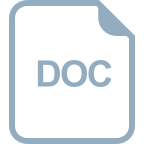
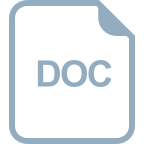
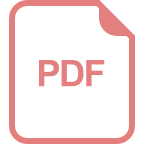
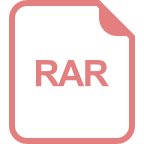
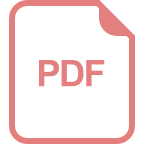
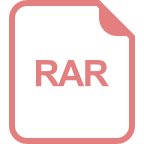
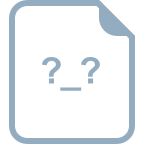
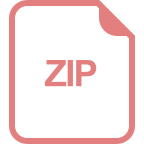
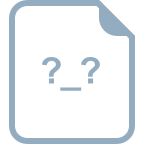
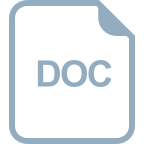