linreg.predict用法
时间: 2024-05-11 18:13:52 浏览: 6
linreg.predict是用于对新数据进行预测的函数。它需要一个参数X,该参数是一个二维数组,表示用于预测的新数据集。
例如,如果我们有一个线性回归模型linreg,我们可以使用如下代码对新数据进行预测:
```python
import numpy as np
# 假设我们有一个新数据集X_new,其中有5个样本,每个样本有3个特征
X_new = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12], [13, 14, 15]])
# 使用linreg模型对X_new进行预测
y_pred = linreg.predict(X_new)
# 打印预测结果
print(y_pred)
```
输出结果将是一个一维数组,包含5个预测值,对应于X_new数据集中的5个样本。
相关问题
解释一下这行代码pred_y = linreg.predict(x)
这行代码使用了一个名为 `linreg` 的线性回归模型对输入数据 `x` 进行预测,并将预测结果存储在 `pred_y` 中。具体解释如下:
- `linreg` 是一个线性回归模型,它已经在代码的其他地方定义并训练好了。
- `predict` 是线性回归模型中的一个方法,用于对给定的输入数据进行预测。
- `x` 是输入的数据,可以是一个数组、列表或其他形式的数据结构。
- `pred_y` 是预测结果,它是一个数组或列表,与输入数据 `x` 对应。
通过这行代码,使用线性回归模型 `linreg` 对输入数据 `x` 进行预测,并将预测结果存储在 `pred_y` 中供后续使用。
self.predict_y = self.reg.predict(_X) AttributeError: 'NoneType' object has no attribute 'predict'
This error message suggests that the object "self.reg" is of type "NoneType", which means it has no attribute called "predict". Therefore, when the code tries to call the "predict" method on "self.reg", it raises an AttributeError.
To fix this error, you need to make sure that "self.reg" is initialized properly and is not None. You can check if "self.reg" is None by adding a print statement before the line that raises the error:
```
print(self.reg) # add this line to check if self.reg is None
self.predict_y = self.reg.predict(_X)
```
If the output of the print statement is "None", then you need to initialize "self.reg" before calling the "predict" method. For example, if you are using scikit-learn's linear regression model, you can initialize "self.reg" as follows:
```
from sklearn.linear_model import LinearRegression
class MyModel:
def __init__(self):
self.reg = LinearRegression()
def fit(self, X, y):
self.reg.fit(X, y)
def predict(self, X):
_X = self._transform(X)
self.predict_y = self.reg.predict(_X)
return self.predict_y
```
This initializes "self.reg" to a new instance of the LinearRegression class, which has the "predict" method you need to call later.
相关推荐
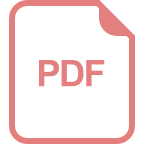
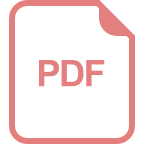
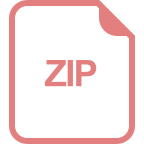












