输入 第一行输入需要进行的解密/加密操作,0表示解密,1表示加密 第二行给出一个以回车结束的非空字符串(少于80个字符) (密文或明文) 第三行输入一个整数offset。 输出 输出加密/解密后的结果字符串。 用C语言编写
时间: 2024-03-14 12:45:59 浏览: 83
以下是一个简单的 C 语言代码,用于对字符串进行加密和解密操作:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 80
void encrypt(char *s, int offset);
void decrypt(char *s, int offset);
int main()
{
int operation;
char s[MAX_LEN + 1];
int offset;
printf("请输入操作 (0表示解密,1表示加密): ");
scanf("%d", &operation);
printf("请输入明文或密文: ");
scanf("%s", s);
printf("请输入偏移量 (整数): ");
scanf("%d", &offset);
if (operation == 0) {
decrypt(s, offset);
printf("解密后的结果为: %s\n", s);
} else if (operation == 1) {
encrypt(s, offset);
printf("加密后的结果为: %s\n", s);
} else {
printf("无效的操作!\n");
}
return 0;
}
void encrypt(char *s, int offset)
{
int i;
for (i = 0; s[i] != '\0'; i++) {
if (s[i] >= 'a' && s[i] <= 'z') {
s[i] = 'a' + (s[i] - 'a' + offset) % 26;
} else if (s[i] >= 'A' && s[i] <= 'Z') {
s[i] = 'A' + (s[i] - 'A' + offset) % 26;
}
}
}
void decrypt(char *s, int offset)
{
int i;
for (i = 0; s[i] != '\0'; i++) {
if (s[i] >= 'a' && s[i] <= 'z') {
s[i] = 'a' + (s[i] - 'a' - offset + 26) % 26;
} else if (s[i] >= 'A' && s[i] <= 'Z') {
s[i] = 'A' + (s[i] - 'A' - offset + 26) % 26;
}
}
}
```
该代码中,我们使用了两个函数 `encrypt` 和 `decrypt` 来进行加密和解密操作。这两个函数都接受一个字符串和一个整数偏移量作为参数。加密函数将字符串中的每个字母向后偏移指定的偏移量,而解密函数则将每个字母向前偏移指定的偏移量,以得到原始的明文。
在 `main` 函数中,我们首先提示用户输入操作 (0 表示解密,1 表示加密),然后读入待处理的字符串和偏移量。接下来,我们根据用户的选择调用相应的函数,并输出结果。
阅读全文
相关推荐
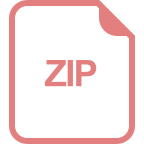




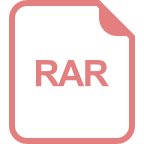
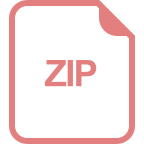
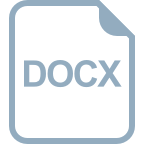
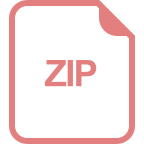