如果数组非常大,有没有更高效的算法来寻找最小值?
时间: 2024-10-25 18:04:19 浏览: 10
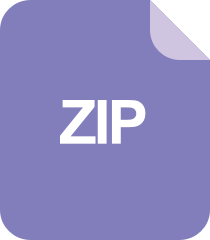
C语言用分治法找数组最大和最小元素算法实现
对于大型数组,查找最小值的一种高效算法是使用分治法或者迭代器。这里有一个基于堆(Heap)的数据结构实现,如优先队列(最小堆)。在最坏的情况下,时间复杂度可以达到O(n log k),其中n是数组长度,k是要找的最小值的数量。如果只需要找到一个最小值,k通常设置为1,此时时间复杂度就是O(n)。
以下是使用PHP和PHP内置的SplDoublyLinkedList(双链表)作为堆的例子,因为它支持插入和删除操作:
```php
class MinHeap {
private $heap;
public function __construct() {
$this->heap = new SplDoublyLinkedList();
}
// 插入元素并保持堆特性
public function insert($value) {
$node = new SplDoublyLinkedListNode($value);
$this->heap->push($node);
while (($parent = $node->prev()) !== null && $value < $parent->data) {
$parent->prev()->next = $node;
$node->prev()->prev = $parent;
$node = $parent;
}
}
// 返回并移除最小值
public function extractMin() {
$minNode = $this->heap->top();
if ($this->heap->count() > 1) {
$last = $this->heap->end();
$last->prev()->next = null;
$this->heap->rewind();
$last->prev()->prev = $last;
} else {
$this->heap = null;
}
return $minNode->data;
}
}
function findMinimumLargeArray($array) {
$heap = new MinHeap();
foreach ($array as $num) {
$heap->insert($num);
}
return $heap->extractMin();
}
// 使用示例
$largeArray = range(1, 100000); // 一个假设的大数组
$minimum = findMinimumLargeArray($largeArray);
```
阅读全文
相关推荐
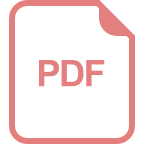
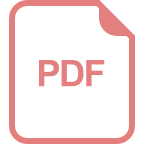
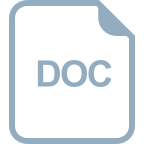
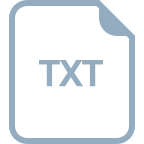
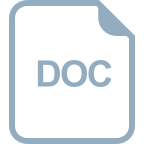
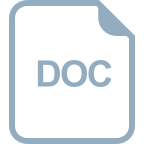
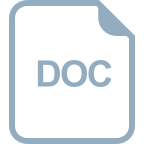
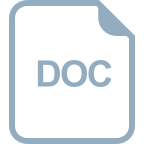
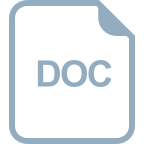
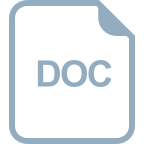
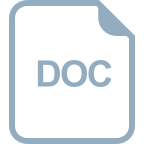
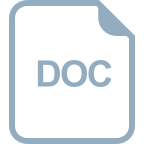
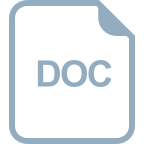
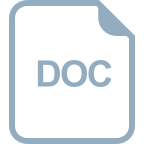
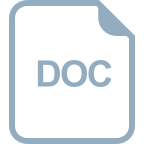
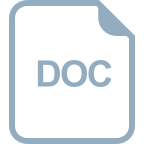
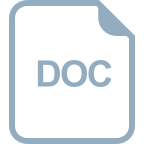