C# web三层架构 实现选择文件的导出路径并导出为excel
时间: 2024-03-26 22:35:09 浏览: 16
首先,你需要在前端页面上添加一个文件选择框,让用户选择要导出的文件。然后,将文件路径传递到后台,进行处理和导出。
在后台,你需要实现以下步骤:
1. 从前端获取文件路径
```csharp
string filePath = Request.Form["filePath"];
```
2. 将数据导出为 Excel 文件
```csharp
//创建Excel文件对象
ExcelPackage excel = new ExcelPackage();
//创建工作表
var sheet = excel.Workbook.Worksheets.Add("Sheet1");
//设置表头
sheet.Cells[1, 1].Value = "姓名";
sheet.Cells[1, 2].Value = "年龄";
//填充数据
sheet.Cells[2, 1].Value = "张三";
sheet.Cells[2, 2].Value = 18;
sheet.Cells[3, 1].Value = "李四";
sheet.Cells[3, 2].Value = 20;
//保存文件
string fileName = "export.xlsx";
string savePath = filePath + "\\" + fileName;
FileInfo file = new FileInfo(savePath);
excel.SaveAs(file);
```
3. 返回文件下载链接
```csharp
Response.Clear();
Response.ContentType = "application/octet-stream";
Response.AppendHeader("Content-Disposition", "attachment;filename=" + fileName);
Response.TransmitFile(savePath);
Response.End();
```
完整的代码示例:
```csharp
public ActionResult ExportExcel()
{
string filePath = Request.Form["filePath"];
if (string.IsNullOrEmpty(filePath))
{
return Content("请选择导出路径!");
}
//创建Excel文件对象
ExcelPackage excel = new ExcelPackage();
//创建工作表
var sheet = excel.Workbook.Worksheets.Add("Sheet1");
//设置表头
sheet.Cells[1, 1].Value = "姓名";
sheet.Cells[1, 2].Value = "年龄";
//填充数据
sheet.Cells[2, 1].Value = "张三";
sheet.Cells[2, 2].Value = 18;
sheet.Cells[3, 1].Value = "李四";
sheet.Cells[3, 2].Value = 20;
//保存文件
string fileName = "export.xlsx";
string savePath = filePath + "\\" + fileName;
FileInfo file = new FileInfo(savePath);
excel.SaveAs(file);
//返回文件下载链接
Response.Clear();
Response.ContentType = "application/octet-stream";
Response.AppendHeader("Content-Disposition", "attachment;filename=" + fileName);
Response.TransmitFile(savePath);
Response.End();
return Content("导出成功!");
}
```
相关推荐
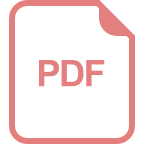














