canvas在舞台上点击后图片旋转_javascript canvas 如何对图片进行绕中心点旋转
时间: 2023-08-11 15:43:48 浏览: 53
可以使用 `translate` 方法将画布的原点移到图片的中心点,然后再使用 `rotate` 方法旋转图片,最后再将画布的原点移回到左上角。
下面是一个示例代码:
```javascript
var canvas = document.getElementById('canvas');
var ctx = canvas.getContext('2d');
var img = new Image();
img.onload = function() {
// 将画布的原点移到图片的中心点
ctx.translate(canvas.width / 2, canvas.height / 2);
// 旋转图片
ctx.rotate(Math.PI / 4);
// 将画布的原点移回到左上角
ctx.translate(-canvas.width / 2, -canvas.height / 2);
// 在画布上绘制图片
ctx.drawImage(img, 0, 0);
};
img.src = 'path/to/image';
```
在上面的示例中,图片被绕着画布中心点旋转了 π/4 弧度。你可以根据需要修改旋转角度。
相关问题
如何用canvas实现一张图片绕画布中心点自动旋转
你可以遵循以下步骤使用 canvas 实现图片绕画布中心点自动旋转:
1. 获取 canvas 元素和 2D 上下文。
2. 创建一个 Image 对象,设置它的 src 属性为你想要旋转的图片地址。
3. 在 Image 对象的 onload 事件中,设置画布的中心点,然后使用 setInterval() 方法以一定的时间间隔重绘画布。
4. 在重绘画布时,先清除画布,然后将画布中心点设置为旋转中心点,使用 translate() 方法将画布移动到旋转中心点处,使用 rotate() 方法旋转画布,最后使用 drawImage() 方法将图片绘制在画布上。
以下是一个示例代码:
```javascript
// 获取 canvas 元素和 2D 上下文
var canvas = document.getElementById('canvas');
var ctx = canvas.getContext('2d');
// 创建 Image 对象
var img = new Image();
img.src = 'image.jpg';
// 在 Image 对象的 onload 事件中,设置画布的中心点和重绘画布
img.onload = function() {
var centerX = canvas.width / 2;
var centerY = canvas.height / 2;
var angle = 0;
setInterval(function() {
// 清除画布
ctx.clearRect(0, 0, canvas.width, canvas.height);
// 将画布移动到旋转中心点处
ctx.translate(centerX, centerY);
// 旋转画布
ctx.rotate(angle * Math.PI / 180);
// 将图片绘制在画布上
ctx.drawImage(img, -img.width / 2, -img.height / 2);
// 恢复画布状态
ctx.rotate(-angle * Math.PI / 180);
ctx.translate(-centerX, -centerY);
// 更新角度
angle += 1;
}, 10);
};
```
这段代码将每 10 毫秒重绘画布一次,并以每次 1 度的速度旋转画布,从而实现了图片绕画布中心点自动旋转的效果。
如何用canvas实现一张图片绕尺寸为800*700居中的画布的中心点自动旋转
可以使用以下步骤来实现:
1. 获取画布和图片的元素,并计算出图片的中心点坐标。
```javascript
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d');
const img = document.getElementById('img');
const canvasWidth = 800;
const canvasHeight = 700;
const imgWidth = img.width;
const imgHeight = img.height;
const imgX = canvasWidth / 2;
const imgY = canvasHeight / 2;
```
2. 设置画布的宽度和高度,并将图片绘制在画布的中心点。
```javascript
canvas.width = canvasWidth;
canvas.height = canvasHeight;
ctx.translate(imgX, imgY);
ctx.drawImage(img, -imgWidth / 2, -imgHeight / 2, imgWidth, imgHeight);
```
3. 使用`requestAnimationFrame`函数来更新图片的旋转角度,并将旋转后的图片绘制到画布上。
```javascript
let angle = 0;
function draw() {
angle += 0.01;
ctx.clearRect(-canvasWidth / 2, -canvasHeight / 2, canvasWidth, canvasHeight);
ctx.rotate(angle);
ctx.drawImage(img, -imgWidth / 2, -imgHeight / 2, imgWidth, imgHeight);
requestAnimationFrame(draw);
}
draw();
```
完整的代码如下:
```javascript
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d');
const img = document.getElementById('img');
const canvasWidth = 800;
const canvasHeight = 700;
const imgWidth = img.width;
const imgHeight = img.height;
const imgX = canvasWidth / 2;
const imgY = canvasHeight / 2;
canvas.width = canvasWidth;
canvas.height = canvasHeight;
ctx.translate(imgX, imgY);
ctx.drawImage(img, -imgWidth / 2, -imgHeight / 2, imgWidth, imgHeight);
let angle = 0;
function draw() {
angle += 0.01;
ctx.clearRect(-canvasWidth / 2, -canvasHeight / 2, canvasWidth, canvasHeight);
ctx.rotate(angle);
ctx.drawImage(img, -imgWidth / 2, -imgHeight / 2, imgWidth, imgHeight);
requestAnimationFrame(draw);
}
draw();
```
相关推荐
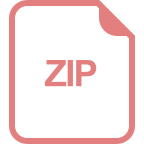
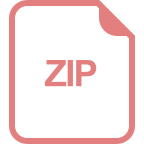












