使用shm_ptr和mmap映射一段匿名共享内存,并向共享内存中拷贝结构体,C语言
时间: 2024-05-06 15:18:56 浏览: 84
以下是一段示例代码,用于使用shm_ptr和mmap映射一段匿名共享内存,并向共享内存中拷贝结构体:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/mman.h>
#include <fcntl.h>
#include <unistd.h>
typedef struct {
int a;
char b[10];
} my_struct;
int main() {
int fd;
my_struct *ptr;
// 创建一个匿名共享内存对象
fd = shm_open("/myshm", O_RDWR | O_CREAT | O_EXCL, 0666);
if (fd == -1) {
perror("shm_open");
exit(1);
}
// 设置共享内存对象的大小为结构体的大小
if (ftruncate(fd, sizeof(my_struct)) == -1) {
perror("ftruncate");
exit(1);
}
// 将共享内存对象映射到进程地址空间中
ptr = (my_struct *)mmap(NULL, sizeof(my_struct), PROT_READ | PROT_WRITE, MAP_SHARED, fd, 0);
if (ptr == MAP_FAILED) {
perror("mmap");
exit(1);
}
// 向共享内存中拷贝结构体
my_struct data = {10, "hello"};
*ptr = data;
// 打印共享内存中的数据
printf("a=%d, b=%s\n", ptr->a, ptr->b);
// 解除共享内存的映射
if (munmap(ptr, sizeof(my_struct)) == -1) {
perror("munmap");
exit(1);
}
// 关闭共享内存对象
if (close(fd) == -1) {
perror("close");
exit(1);
}
// 移除共享内存对象
if (shm_unlink("/myshm") == -1) {
perror("shm_unlink");
exit(1);
}
return 0;
}
```
注意,以上代码需要在Linux下编译运行,因为Windows不支持shm_ptr和mmap。另外,需要注意多进程之间对共享内存的访问顺序和同步问题,避免出现数据不一致的情况。
阅读全文
相关推荐
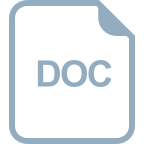
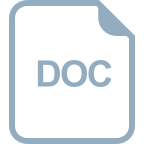
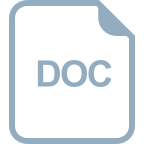
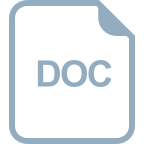
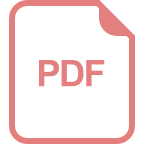
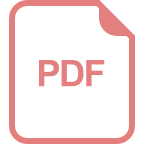
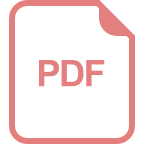
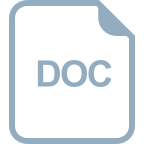
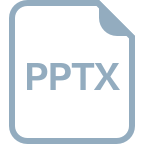
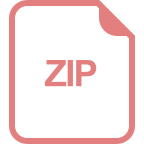
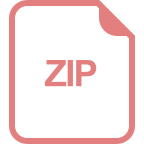
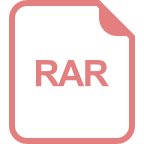
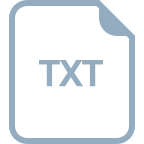
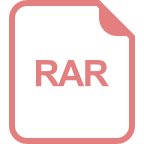
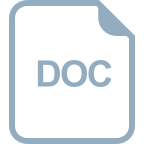
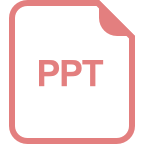
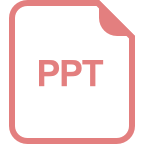
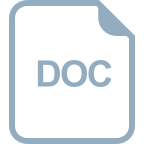
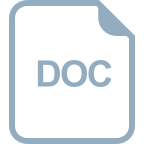