qt qinputdialog自定义
时间: 2023-10-24 14:09:20 浏览: 190
输入框怎么设置默认值?
对于自定义QInputDialog输入框设置默认值,可以使用QInputDialog::setTextValue函数来进行设置。示例如下:
```
QInputDialog dialog;
dialog.setInputMode(QInputDialog::TextInput);
dialog.setTextValue("default value");
dialog.exec();
```
这段代码会打开一个只有一个文本输入框的QInputDialog对话框,文本输入框中默认值为"default value"。
注意:QInputDialog::setTextValue函数只能在对话框显示之前调用才会生效。
相关问题
qt c++ 自定义树模型 模型flags 并未设置为ItemIsEditable, 右击菜单 建立重命名QAction
好的,您的问题是关于在自定义树模型中实现右键菜单的重命名功能。首先,您需要在自定义树模型中实现 `flags()` 函数,将 `Qt::ItemIsEditable` 添加到返回值中,以允许项目可编辑。这样用户就可以编辑项目了。
其次,您需要创建一个右键菜单,并在其中添加一个重命名的 `QAction`。在 `QAction` 上连接一个槽函数,以响应用户在菜单中选择重命名的操作。
在槽函数中,您可以使用 `QModelIndex` 获取到用户选择的项目的索引。然后,使用 `setData()` 函数将新的项目名称设置为该项目的数据。最后,调用 `dataChanged()` 函数来更新该项目的视图。
下面是一些示例代码,用于演示如何实现这个功能:
```cpp
Qt::ItemFlags YourTreeModel::flags(const QModelIndex& index) const
{
Qt::ItemFlags flags = QAbstractItemModel::flags(index);
flags |= Qt::ItemIsEditable;
return flags;
}
void YourTreeModel::onCustomContextMenuRequested(const QPoint& pos)
{
QModelIndex index = indexAt(pos);
if (!index.isValid()) {
return;
}
QMenu menu;
QAction* renameAction = menu.addAction(tr("Rename"));
QAction* selectedItem = menu.exec(viewport()->mapToGlobal(pos));
if (selectedItem == renameAction) {
renameItem(index);
}
}
void YourTreeModel::renameItem(const QModelIndex& index)
{
QString oldName = data(index, Qt::DisplayRole).toString();
bool ok;
QString newName = QInputDialog::getText(nullptr, tr("Rename Item"), tr("New Name:"), QLineEdit::Normal, oldName, &ok);
if (ok && !newName.isEmpty()) {
setData(index, newName, Qt::DisplayRole);
emit dataChanged(index, index);
}
}
```
您需要在 `YourTreeModel` 类中实现 `onCustomContextMenuRequested()` 和 `renameItem()` 函数,用于处理右键菜单事件和重命名操作。在 `flags()` 函数中,将 `Qt::ItemIsEditable` 标志添加到项目的标志中。
希望这可以帮助您实现所需的功能!
qt 同一个窗口多个QInputDialog
如果要在同一个窗口中创建多个QInputDialog,可以使用QDialog类来创建自定义的输入对话框。您可以为每个对话框创建一个单独的QDialog,并在需要时显示它们。
以下是一个简单的示例,演示如何创建两个自定义输入对话框并在同一个窗口中显示它们:
```
#include <QDialog>
#include <QLineEdit>
#include <QLabel>
#include <QVBoxLayout>
#include <QPushButton>
class InputDialog : public QDialog
{
Q_OBJECT
public:
explicit InputDialog(const QString& title, const QString& label, QWidget *parent = nullptr)
: QDialog(parent)
{
setWindowTitle(title);
QLabel* inputLabel = new QLabel(label, this);
m_input = new QLineEdit(this);
QVBoxLayout* layout = new QVBoxLayout(this);
layout->addWidget(inputLabel);
layout->addWidget(m_input);
QPushButton* okButton = new QPushButton(tr("OK"), this);
connect(okButton, &QPushButton::clicked, this, &QDialog::accept);
layout->addWidget(okButton);
}
QString text() const
{
return m_input->text();
}
private:
QLineEdit* m_input;
};
void MyWidget::showInputDialogs()
{
InputDialog dialog1(tr("Input Dialog 1"), tr("Enter some text:"), this);
InputDialog dialog2(tr("Input Dialog 2"), tr("Enter some text:"), this);
if (dialog1.exec() == QDialog::Accepted) {
QString text1 = dialog1.text();
// do something with text1
}
if (dialog2.exec() == QDialog::Accepted) {
QString text2 = dialog2.text();
// do something with text2
}
}
```
在这个例子中,我们创建了一个名为InputDialog的自定义对话框类,它包含一个标签和一个文本框以用于输入。我们在MyWidget类中创建了两个InputDialog实例,并在需要时显示它们。在用户关闭对话框时,我们可以使用text()函数来检索输入的文本。
阅读全文
相关推荐
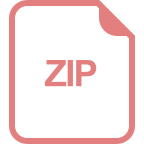
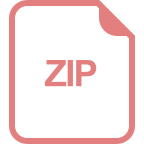
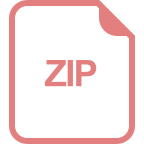
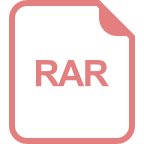
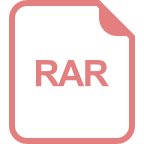
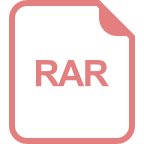
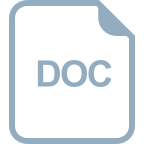
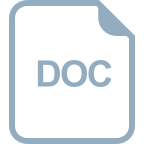







