springboot实现文件断点上传代码
时间: 2023-12-20 07:07:28 浏览: 85
Spring Boot 实现文件断点上传可以使用 MultipartFile 类,配合 RandomAccessFile 类和 Range 头部实现。以下是一个简单的示例代码:
```java
@RestController
public class FileController {
private final String UPLOAD_FOLDER = "/path/to/upload/folder/";
@PostMapping("/upload")
public ResponseEntity<?> uploadFile(@RequestParam("file") MultipartFile multipartFile,
@RequestHeader(value = "Range", required = false) String rangeHeader) {
try {
File file = new File(UPLOAD_FOLDER + multipartFile.getOriginalFilename());
// If Range header is present, it means we are resuming an upload
if (rangeHeader != null) {
long range = Long.parseLong(rangeHeader.replace("bytes=", "").replace("-", ""));
RandomAccessFile randomAccessFile = new RandomAccessFile(file, "rw");
randomAccessFile.seek(range);
randomAccessFile.write(multipartFile.getBytes());
randomAccessFile.close();
} else {
multipartFile.transferTo(file);
}
return ResponseEntity.ok("File uploaded successfully!");
} catch (IOException e) {
e.printStackTrace();
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("Failed to upload file!");
}
}
}
```
在上传文件时,如果请求头中包含 Range 头部,则说明需要断点续传,此时需要使用 RandomAccessFile 类来定位文件指针,并写入上传的文件块。如果 Range 头部不存在,则直接使用 MultipartFile.transferTo() 方法上传整个文件。
阅读全文
相关推荐
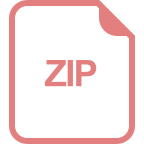
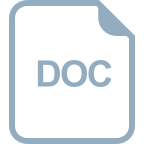

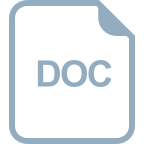
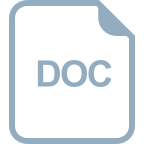
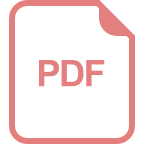
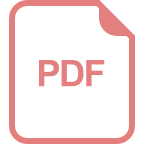
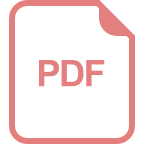
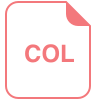




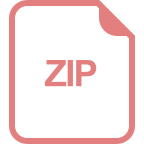
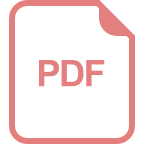