请用【java】帮我写一段图书管理系统的代码
时间: 2024-10-17 12:12:56 浏览: 25
在Java中,创建一个简单的图书管理系统可以包含几个类,如图书、图书分类和图书馆。这里是一个基础的示例,我们将展示如何设计基本的概念:
```java
// 定义图书类Book
public class Book {
private String title;
private String author;
private String category;
// 构造函数和getter/setter
public Book(String title, String author, String category) {
this.title = title;
this.author = author;
this.category = category;
}
public String getTitle() { return title; }
public void setTitle(String title) { this.title = title; }
public String getAuthor() { return author; }
public void setAuthor(String author) { this.author = author; }
public String getCategory() { return category; }
public void setCategory(String category) { this.category = category; }
}
// 定义图书分类类Category
public class Category {
private String name;
// 构造函数和getter/setter
public Category(String name) {
this.name = name;
}
public String getName() { return name; }
public void setName(String name) { this.name = name; }
}
// 定义图书馆类Library
import java.util.ArrayList;
import java.util.List;
public class Library {
private List<Book> books;
public Library() {
this.books = new ArrayList<>();
}
// 添加书籍到图书馆
public void addBook(Book book) {
books.add(book);
}
// 按照分类查找书籍
public List<Book> findBooksByCategory(Category category) {
List<Book> result = new ArrayList<>();
for (Book book : books) {
if (book.getCategory().equals(category.getName())) {
result.add(book);
}
}
return result;
}
}
```
这只是一个非常基础的示例,实际应用中可能会有借阅历史、用户权限控制等功能。在这个例子中,你可以通过`Library`实例添加书并按类别查询。
阅读全文
相关推荐













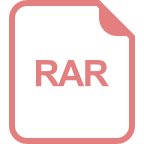


