前端数组对象树结构扁平化
时间: 2024-09-11 19:03:29 浏览: 34
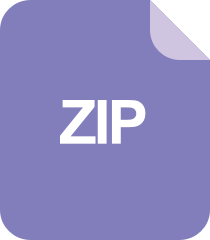
js代码-数组拍平,扁平化 flat
前端数组对象树结构扁平化是指将嵌套的对象数组转换成一维的、简单的键值对数组。这种操作通常发生在需要处理数据展示或API请求返回的数据,以便更容易地遍历和操作。例如,假设你有这样一个树状结构:
```json
{
"id": 1,
"name": "Root",
"children": [
{
"id": 2,
"name": "Child 1",
"children": [
{"id": 4, "name": "Grandchild 1"},
{"id": 5, "name": "Grandchild 2"}
]
},
{
"id": 3,
"name": "Child 2"
}
]
}
```
扁平化后可能会变成:
```json
[
{ id: 1, name: 'Root' },
{ id: 2, name: 'Child 1', children: [{ id: 4, name: 'Grandchild 1' }, { id: 5, name: 'Grandchild 2' }] },
{ id: 3, name: 'Child 2' }
]
```
这个过程可以使用递归或循环的方式实现,JavaScript中常见的库如lodash或手写的for循环都能完成这样的任务。
阅读全文
相关推荐
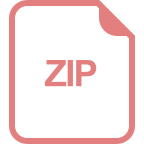
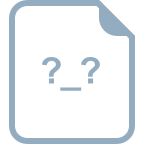
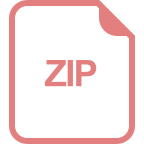
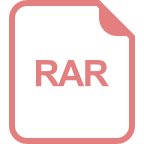
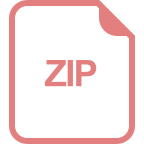
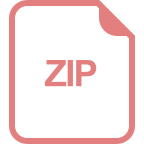
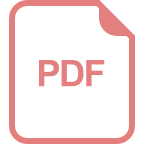
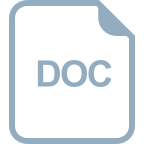
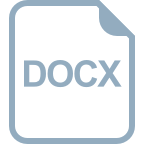
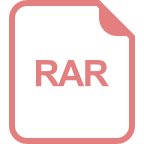
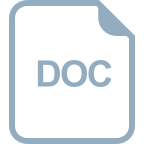
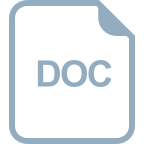
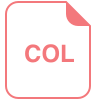
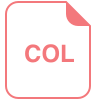
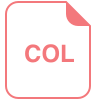
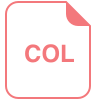
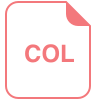