String s="我"; byte[] bytes = s.getBytes(StandardCharsets.UTF_8); FileReader fileReader = new FileReader("C:\\Users\\36924\\IdeaProjects\\basic-code\\MyFile-IO\\src\\byte_stream\\book"); int read = fileReader.read(); System.out.println(read);
时间: 2023-08-27 22:21:56 浏览: 53
这段代码的作用是读取文件中的一个字节,并将其打印出来。具体来说,首先定义了一个字符串变量s,并将其转换成UTF-8编码的字节数组。然后创建一个FileReader对象,用于读取文件。接着调用FileReader对象的read()方法读取文件中的一个字节,并将其赋值给变量read。最后将变量read打印出来。需要注意的是,这段代码只读取了文件中的一个字节,并没有对文件内容进行完整读取。
相关问题
import java.io.*; public class TestCopy1 { private static void createStuFile() throws IOException { File file = new File("fin.txt"); FileOutputStream fos = new FileOutputStream(file); String str = "Alcatel provides end-to-end solutions."; byte[] m = str.getBytes(); fos.write(m); fos.close(); } public static void main(String[] args) throws IOException { createStuFile(); File file = new File("fin.txt"); File file2 =new File("fout.txt"); FileReader fr = new FileReader(file); FileWriter fw = new FileWriter(file2); int x = fr.read(); while(x!=-1) { if((char)x==' '||(char)x=='\t') { x=(char)x; x.replaceAll(" +", " "); } System.out.print((char)x); fw.write(x); x = fr.read(); } fr.close(); fw.close(); } }代码改错
代码中有一个错误:在将字符替换为空格时,使用了 `replaceAll()` 方法,但该方法是 String 类的方法,不能直接应用于字符变量。应该改为使用字符常量 `' '` 来表示空格,并使用 `Character.toString()` 方法将其转换为字符串:
```
if ((char) x == ' ' || (char) x == '\t') {
x = ' ';
String space = Character.toString((char) x);
str = str.replaceAll(" +" + space, space);
}
```
另外,代码中的 `fw.write(x)` 应该是 `fw.write((char) x)`,否则写出的是字节而非字符。完整代码如下:
```java
import java.io.*;
public class TestCopy1 {
private static void createStuFile() throws IOException {
File file = new File("fin.txt");
FileOutputStream fos = new FileOutputStream(file);
String str = "Alcatel provides end-to-end solutions.";
byte[] m = str.getBytes();
fos.write(m);
fos.close();
}
public static void main(String[] args) throws IOException {
createStuFile();
File file = new File("fin.txt");
File file2 = new File("fout.txt");
FileReader fr = new FileReader(file);
FileWriter fw = new FileWriter(file2);
int x = fr.read();
String str = "";
while (x != -1) {
if ((char) x == ' ' || (char) x == '\t') {
x = ' ';
String space = Character.toString((char) x);
str = str.replaceAll(" +" + space, space);
}
str += (char) x;
System.out.print((char) x);
fw.write((char) x);
x = fr.read();
}
System.out.println("\n\n" + str); // 打印替换后的字符串
fr.close();
fw.close();
}
}
```
在程序中定义一字符串”hello,how are you!”,分别用字节流和字符流实现将该字符串保存到d:\t2_1.txt, d:\t2_2.txt中,程序分别保存为Test2_1.java和Test2_2.java。
好的,这是一份使用Java语言实现的代码示例,可以实现您的需求:
```java
import java.io.*;
public class Test2_1 {
public static void main(String[] args) {
String str = "hello,how are you!";
// 使用字节流保存字符串到d:\t2_1.txt
try (FileOutputStream fos = new FileOutputStream("d:\\t2_1.txt")) {
byte[] bytes = str.getBytes();
fos.write(bytes);
System.out.println("保存到d:\\t2_1.txt成功!");
} catch (IOException e) {
System.out.println("保存到d:\\t2_1.txt失败!" + e.getMessage());
}
// 使用字符流保存字符串到d:\t2_2.txt
try (FileWriter fw = new FileWriter("d:\\t2_2.txt")) {
fw.write(str);
System.out.println("保存到d:\\t2_2.txt成功!");
} catch (IOException e) {
System.out.println("保存到d:\\t2_2.txt失败!" + e.getMessage());
}
}
}
```
```java
import java.io.*;
public class Test2_2 {
public static void main(String[] args) {
// 从d:\t2_1.txt读取字节流并保存为字符串
try (FileInputStream fis = new FileInputStream("d:\\t2_1.txt")) {
byte[] bytes = new byte[fis.available()];
fis.read(bytes);
String str = new String(bytes);
System.out.println("从d:\\t2_1.txt读取的字符串为:" + str);
} catch (IOException e) {
System.out.println("从d:\\t2_1.txt读取字符串失败!" + e.getMessage());
}
// 从d:\t2_2.txt读取字符流并保存为字符串
try (FileReader fr = new FileReader("d:\\t2_2.txt")) {
char[] chars = new char[1024];
StringBuilder sb = new StringBuilder();
int len;
while ((len = fr.read(chars)) != -1) {
sb.append(chars, 0, len);
}
String str = sb.toString();
System.out.println("从d:\\t2_2.txt读取的字符串为:" + str);
} catch (IOException e) {
System.out.println("从d:\\t2_2.txt读取字符串失败!" + e.getMessage());
}
}
}
```
相关推荐
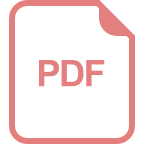
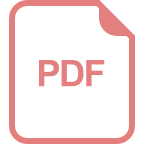
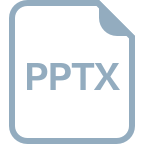












