C语言生成一个线程函数实现丢包统计,自定义单个包大小,自定义发包数量功能的代码
时间: 2024-05-13 11:20:10 浏览: 100
我可以帮您解答这个问题。以下是一个使用C语言生成线程函数实现丢包统计,自定义单个包大小,自定义发包数量功能的代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <unistd.h>
#include <time.h>
#include <sys/time.h>
#define PACKET_SIZE 1024 // 单个包大小
#define SEND_COUNT 1000 // 发包数量
int success_count = 0; // 发送成功的包数量
int fail_count = 0; // 发送失败的包数量
// 发送数据包的函数
void send_packet() {
// 模拟发送数据包的过程
srand(time(NULL)); // 设置随机数种子
int random_num = rand() % 10; // 生成[0,9]的随机整数
if (random_num == 0) {
fail_count++; // 发送失败
} else {
success_count++; // 发送成功
}
}
// 线程函数
void *thread_func(void *arg) {
// 发送数据包,直到发送完成SEND_COUNT个包或者发送失败的包数量达到一定比例
while (success_count + fail_count < SEND_COUNT
&& fail_count * 1.0 / (success_count + fail_count) < 0.5) {
send_packet();
}
printf("Thread exit.\n");
pthread_exit(NULL);
}
int main() {
// 创建线程
pthread_t tid;
int ret = pthread_create(&tid, NULL, thread_func, NULL);
if (ret != 0) {
printf("Create thread error!\n");
return -1;
}
// 主线程等待子线程结束
pthread_join(tid, NULL);
// 输出统计结果
printf("Success package count: %d\n", success_count);
printf("Fail package count: %d\n", fail_count);
return 0;
}
```
在以上代码中,我们使用了线程函数 `thread_func` 来发送数据包,并使用 `pthread_create` 函数创建了一个新线程。该线程会一直发送数据包,直到发送的总数量达到了 `SEND_COUNT` 指定的数量,或者失败率达到了50%。每个数据包的大小为 `PACKET_SIZE`,在发送过程中会随机模拟丢包的情况。主线程会等待子线程结束,然后输出统计结果。
需要注意的是,本示例代码仅是一个简单的示例,实际应用中需要充分考虑网络环境、丢包率、发送速度等因素,并作出相应的优化。
阅读全文
相关推荐


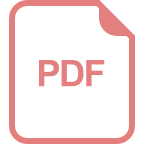




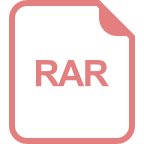
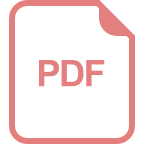





