cla = name[1] size = name[2]
时间: 2023-11-26 08:03:38 浏览: 19
这段代码片段是在 Python 中使用索引访问列表中的元素。
假设 `name` 是一个列表,那么 `name[1]` 表示访问列表中的第二个元素(列表的索引从0开始),将其赋值给变量 `cla`。
同理,`name[2]` 表示访问列表中的第三个元素,将其赋值给变量 `size`。
需要注意的是,如果 `name` 列表的长度小于 3,那么访问 `name[2]` 会导致 `IndexError` 异常。
相关问题
这是对单个文件进行预测“import os import json import torch from PIL import Image from torchvision import transforms import matplotlib.pyplot as plt from model import convnext_tiny as create_model def main(): device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu") print(f"using {device} device.") num_classes = 5 img_size = 224 data_transform = transforms.Compose( [transforms.Resize(int(img_size * 1.14)), transforms.CenterCrop(img_size), transforms.ToTensor(), transforms.Normalize([0.485, 0.456, 0.406], [0.229, 0.224, 0.225])]) # load image img_path = "../tulip.jpg" assert os.path.exists(img_path), "file: '{}' dose not exist.".format(img_path) img = Image.open(img_path) plt.imshow(img) # [N, C, H, W] img = data_transform(img) # expand batch dimension img = torch.unsqueeze(img, dim=0) # read class_indict json_path = './class_indices.json' assert os.path.exists(json_path), "file: '{}' dose not exist.".format(json_path) with open(json_path, "r") as f: class_indict = json.load(f) # create model model = create_model(num_classes=num_classes).to(device) # load model weights model_weight_path = "./weights/best_model.pth" model.load_state_dict(torch.load(model_weight_path, map_location=device)) model.eval() with torch.no_grad(): # predict class output = torch.squeeze(model(img.to(device))).cpu() predict = torch.softmax(output, dim=0) predict_cla = torch.argmax(predict).numpy() print_res = "class: {} prob: {:.3}".format(class_indict[str(predict_cla)], predict[predict_cla].numpy()) plt.title(print_res) for i in range(len(predict)): print("class: {:10} prob: {:.3}".format(class_indict[str(i)], predict[i].numpy())) plt.show() if name == 'main': main()”,改为对指定文件夹下的左右文件进行预测,并绘制混淆矩阵,
好的,为了实现您的需求,您需要进行以下几个步骤:
1. 加载模型和类别标签:
您需要在代码中加载您的模型和类别标签。您可以使用之前的代码中的方式来加载它们,只需要做一些小的改动即可。例如,您需要将模型和类别标签的路径作为参数传递给您的函数。
2. 加载测试集:
您需要加载您的测试集。您可以使用 `torchvision.datasets.ImageFolder` 来加载测试集。这个函数会将每个文件夹中的所有图像文件都加载到一个 tensor 中,并自动为每个文件夹分配一个标签。
3. 进行预测:
您需要对测试集中的每个图像进行预测,并将预测结果与真实标签进行比较。您可以使用之前的代码中的方式来预测每个图像,只需要做一些小的改动即可。例如,您需要将预测结果保存到一个列表中,并将真实标签保存到另一个列表中。
4. 绘制混淆矩阵:
最后,您需要使用预测结果和真实标签来绘制混淆矩阵。您可以使用 `sklearn.metrics.confusion_matrix` 来计算混淆矩阵,并使用 `matplotlib` 来绘制它。
下面是修改后的代码示例:
```
import os
import json
import torch
from PIL import Image
from torchvision import transforms
import matplotlib.pyplot as plt
from sklearn.metrics import confusion_matrix
import numpy as np
from model import convnext_tiny as create_model
def predict_folder(model_path, json_path, folder_path):
device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu")
print(f"using {device} device.")
num_classes = 5
img_size = 224
data_transform = transforms.Compose([
transforms.Resize(int(img_size * 1.14)),
transforms.CenterCrop(img_size),
transforms.ToTensor(),
transforms.Normalize([0.485, 0.456, 0.406], [0.229, 0.224, 0.225])
])
# load class_indict json
with open(json_path, "r") as f:
class_indict = json.load(f)
# create model
model = create_model(num_classes=num_classes).to(device)
# load model weights
model.load_state_dict(torch.load(model_path, map_location=device))
model.eval()
y_true = []
y_pred = []
for root, dirs, files in os.walk(folder_path):
for file in files:
if file.endswith(".jpg") or file.endswith(".jpeg"):
img_path = os.path.join(root, file)
assert os.path.exists(img_path), "file: '{}' dose not exist.".format(img_path)
img = Image.open(img_path)
# [N, C, H, W]
img = data_transform(img)
# expand batch dimension
img = torch.unsqueeze(img, dim=0)
# predict class
with torch.no_grad():
output = torch.squeeze(model(img.to(device))).cpu()
predict = torch.softmax(output, dim=0)
predict_cla = torch.argmax(predict).numpy()
y_true.append(class_indict[os.path.basename(root)])
y_pred.append(predict_cla)
# plot confusion matrix
cm = confusion_matrix(y_true, y_pred)
fig, ax = plt.subplots(figsize=(5, 5))
ax.imshow(cm, cmap=plt.cm.Blues, aspect='equal')
ax.set_xlabel('Predicted label')
ax.set_ylabel('True label')
ax.set_xticks(np.arange(len(class_indict)))
ax.set_yticks(np.arange(len(class_indict)))
ax.set_xticklabels(class_indict.values(), rotation=90)
ax.set_yticklabels(class_indict.values())
ax.tick_params(axis=u'both', which=u'both',length=0)
for i in range(len(class_indict)):
for j in range(len(class_indict)):
text = ax.text(j, i, cm[i, j], ha="center", va="center", color="white" if cm[i, j] > cm.max() / 2. else "black")
fig.tight_layout()
plt.show()
if __name__ == '__main__':
# set the paths for the model, class_indict json, and test data folder
model_path = './weights/best_model.pth'
json_path = './class_indices.json'
folder_path = './test_data'
predict_folder(model_path, json_path, folder_path)
```
请注意,这个函数的参数需要您自己根据您的实际情况进行设置,以匹配模型、类别标签和测试集的路径。
这是对单个文件进行预测“import os import json import torch from PIL import Image from torchvision import transforms import matplotlib.pyplot as plt from model import convnext_tiny as create_model def main(): device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu") print(f"using {device} device.") num_classes = 5 img_size = 224 data_transform = transforms.Compose( [transforms.Resize(int(img_size * 1.14)), transforms.CenterCrop(img_size), transforms.ToTensor(), transforms.Normalize([0.485, 0.456, 0.406], [0.229, 0.224, 0.225])]) # load image img_path = "../tulip.jpg" assert os.path.exists(img_path), "file: '{}' dose not exist.".format(img_path) img = Image.open(img_path) plt.imshow(img) # [N, C, H, W] img = data_transform(img) # expand batch dimension img = torch.unsqueeze(img, dim=0) # read class_indict json_path = './class_indices.json' assert os.path.exists(json_path), "file: '{}' dose not exist.".format(json_path) with open(json_path, "r") as f: class_indict = json.load(f) # create model model = create_model(num_classes=num_classes).to(device) # load model weights model_weight_path = "./weights/best_model.pth" model.load_state_dict(torch.load(model_weight_path, map_location=device)) model.eval() with torch.no_grad(): # predict class output = torch.squeeze(model(img.to(device))).cpu() predict = torch.softmax(output, dim=0) predict_cla = torch.argmax(predict).numpy() print_res = "class: {} prob: {:.3}".format(class_indict[str(predict_cla)], predict[predict_cla].numpy()) plt.title(print_res) for i in range(len(predict)): print("class: {:10} prob: {:.3}".format(class_indict[str(i)], predict[i].numpy())) plt.show() if __name__ == '__main__': main()”,改为对指定文件夹下的左右文件进行预测,并绘制混淆矩阵
好的,你的问题是如何将上述代码修改为对指定文件夹下的所有文件进行预测,并绘制混淆矩阵。
要实现这个功能,你需要进行以下几个步骤:
1. 导入必要的包:
```python
import os
import json
import torch
from PIL import Image
from torchvision import transforms
from sklearn.metrics import confusion_matrix
import matplotlib.pyplot as plt
import numpy as np
from model import convnext_tiny as create_model
```
2. 定义函数 `predict_folder`,用于对指定文件夹下的所有文件进行预测:
```python
def predict_folder(folder_path, model_weight_path, json_path, img_size, num_classes, device):
data_transform = transforms.Compose([
transforms.Resize(int(img_size * 1.14)),
transforms.CenterCrop(img_size),
transforms.ToTensor(),
transforms.Normalize([0.485, 0.456, 0.406], [0.229, 0.224, 0.225])
])
# read class_indict
with open(json_path, "r") as f:
class_indict = json.load(f)
# create model
model = create_model(num_classes=num_classes).to(device)
# load model weights
model.load_state_dict(torch.load(model_weight_path, map_location=device))
model.eval()
y_true = []
y_pred = []
for root, dirs, files in os.walk(folder_path):
for file in files:
img_path = os.path.join(root, file)
assert os.path.exists(img_path), "file: '{}' dose not exist.".format(img_path)
img = Image.open(img_path)
# [N, C, H, W]
img = data_transform(img)
# expand batch dimension
img = torch.unsqueeze(img, dim=0)
with torch.no_grad():
# predict class
output = torch.squeeze(model(img.to(device))).cpu()
predict = torch.softmax(output, dim=0)
predict_cla = torch.argmax(predict).numpy()
y_true.append(class_indict[os.path.basename(root)])
y_pred.append(predict_cla)
return y_true, y_pred
```
这个函数接受五个参数:
- `folder_path`:要预测的文件夹路径。
- `model_weight_path`:模型权重文件路径。
- `json_path`:类别标签文件路径。
- `img_size`:输入图片的大小。
- `num_classes`:分类器的类别数。
- `device`:设备类型。
函数会返回两个列表 `y_true` 和 `y_pred`,分别代表真实标签和预测标签。
3. 加载类别标签:
```python
json_path = './class_indices.json'
assert os.path.exists(json_path), "file: '{}' dose not exist.".format(json_path)
with open(json_path, "r") as f:
class_indict = json.load(f)
```
4. 调用 `predict_folder` 函数进行预测:
```python
folder_path = './test'
assert os.path.exists(folder_path), "folder: '{}' dose not exist.".format(folder_path)
y_true, y_pred = predict_folder(folder_path, "./weights/best_model.pth", json_path, 224, 5, device)
```
这里假设要预测的文件夹路径为 `./test`,模型权重文件路径为 `./weights/best_model.pth`,输入图片大小为 224,分类器的类别数为 5。
5. 绘制混淆矩阵:
```python
cm = confusion_matrix(y_true, y_pred)
fig, ax = plt.subplots()
im = ax.imshow(cm, interpolation='nearest', cmap=plt.cm.Blues)
ax.figure.colorbar(im, ax=ax)
ax.set(xticks=np.arange(cm.shape[1]),
yticks=np.arange(cm.shape[0]),
xticklabels=list(class_indict.values()), yticklabels=list(class_indict.values()),
title='Confusion matrix',
ylabel='True label',
xlabel='Predicted label')
plt.setp(ax.get_xticklabels(), rotation=45, ha="right",
rotation_mode="anchor")
fmt = 'd'
thresh = cm.max() / 2.
for i in range(cm.shape[0]):
for j in range(cm.shape[1]):
ax.text(j, i, format(cm[i, j], fmt),
ha="center", va="center",
color="white" if cm[i, j] > thresh else "black")
fig.tight_layout()
plt.show()
```
这里使用了 `sklearn.metrics` 中的 `confusion_matrix` 函数进行混淆矩阵的计算。然后使用 `matplotlib` 绘制混淆矩阵图像。
相关推荐
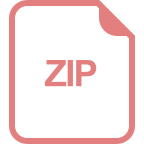












