java简单共享单车程序代码
时间: 2023-08-30 08:04:27 浏览: 131
以下是一个简单的Java共享单车程序的代码示例:
```java
import java.util.ArrayList;
import java.util.List;
public class Bike {
private String id;
private int location;
private boolean isAvailable;
// Constructor
public Bike(String id, int location) {
this.id = id;
this.location = location;
this.isAvailable = true;
}
// Getter and Setter methods
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public int getLocation() {
return location;
}
public void setLocation(int location) {
this.location = location;
}
public boolean isAvailable() {
return isAvailable;
}
public void setAvailable(boolean available) {
isAvailable = available;
}
}
public class BikeSystem {
private List<Bike> bikes;
// Constructor
public BikeSystem() {
this.bikes = new ArrayList<>();
}
// Add a bike to the system
public void addBike(Bike bike) {
bikes.add(bike);
}
// Remove a bike from the system
public void removeBike(Bike bike) {
bikes.remove(bike);
}
// Update the location of a bike
public void updateBikeLocation(Bike bike, int newLocation) {
bike.setLocation(newLocation);
}
// Rent a bike
public Bike rentBike(String id) {
for (Bike bike : bikes) {
if (bike.getId().equals(id) && bike.isAvailable()) {
bike.setAvailable(false);
return bike;
}
}
return null;
}
// Return a bike
public void returnBike(Bike bike) {
bike.setAvailable(true);
}
}
public class Main {
public static void main(String[] args) {
BikeSystem bikeSystem = new BikeSystem();
// Add some bikes to the system
bikeSystem.addBike(new Bike("001", 1));
bikeSystem.addBike(new Bike("002", 2));
bikeSystem.addBike(new Bike("003", 3));
bikeSystem.addBike(new Bike("004", 4));
bikeSystem.addBike(new Bike("005", 5));
// Rent a bike
Bike rentedBike = bikeSystem.rentBike("002");
if (rentedBike != null) {
System.out.println("Bike " + rentedBike.getId() + " is rented.");
} else {
System.out.println("Bike is not available.");
}
// Return the bike
bikeSystem.returnBike(rentedBike);
System.out.println("Bike " + rentedBike.getId() + " is returned.");
}
}
```
在这个示例程序中,`Bike`类表示一辆共享单车,`BikeSystem`类表示共享单车管理系统。通过这两个类的方法,可以实现添加、删除、更新单车信息,租用单车和还车等功能。在`Main`类中,我们创建了一个`BikeSystem`对象,并添加了一些单车到系统中。我们还租用了一辆单车,然后还车,并打印出相应的信息。
阅读全文
相关推荐

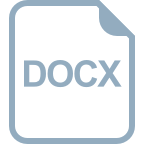
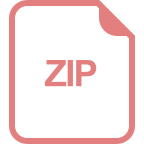
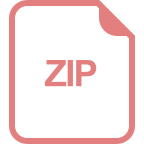
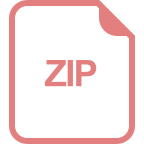
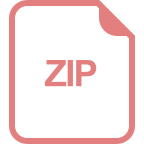
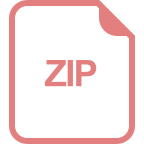
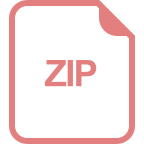
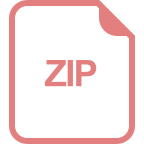
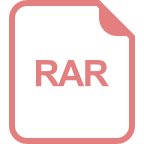
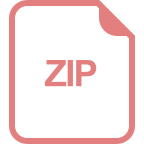
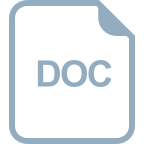
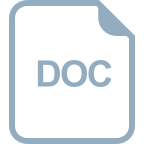
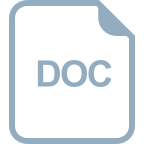
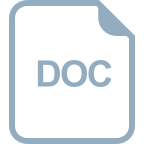
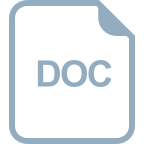