mapreduce设计模式
时间: 2023-12-28 13:26:21 浏览: 148
MapReduce设计模式是一种用于解决大规模数据处理问题的编程模式。它基于分布式计算框架MapReduce,通过将问题分解为多个独立的任务(Map任务和Reduce任务),并在多台计算机上并行执行这些任务,从而实现高效的数据处理。
MapReduce设计模式的核心思想是将输入数据集划分为多个小的数据块,然后对每个数据块进行独立的处理。在Map阶段,每个数据块会被映射为一组键值对,其中键表示数据的某个特征,值表示该特征对应的数据。在Reduce阶段,相同键的数据会被合并在一起,并进行进一步的处理和分析。
通过使用MapReduce设计模式,我们可以解决各种类型的问题,例如计数、排序、过滤、聚合等。具体的设计模式取决于问题的特点和需求。《MapReduce设计模式》这本书提供了一些常见的设计模式,可以作为解决问题的模板或通用指南。
以下是一个简单的示例,演示了如何使用MapReduce设计模式来计算一个文本文件中每个单词的出现次数:
```python
# Map函数:将每个单词映射为键值对(单词, 1)
def map_func(line):
words = line.split()
return [(word, 1) for word in words]
# Reduce函数:对相同单词的计数进行累加
def reduce_func(word, counts):
return (word, sum(counts))
# 输入数据
input_data = [
"Hello world",
"Hello mapreduce",
"MapReduce is powerful"
]
# 执行MapReduce任务
result = {}
for line in input_data:
for word, count in map_func(line):
if word in result:
result[word].append(count)
else:
result[word] = [count]
final_result = [reduce_func(word, counts) for word, counts in result.items()]
# 输出结果
for word, count in final_result:
print(f"{word}: {count}")
```
输出结果:
```
Hello: 2
world: 1
mapreduce: 1
is: 1
powerful: 1
```
阅读全文
相关推荐
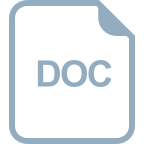
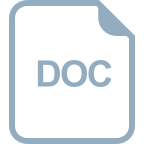
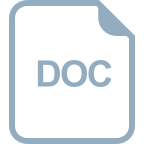
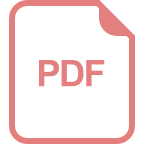
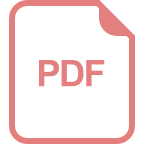
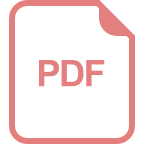
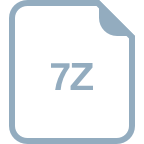
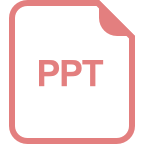

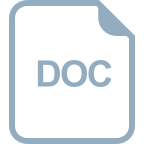
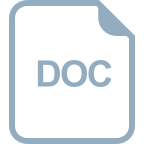
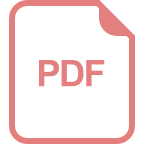
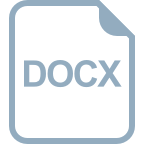
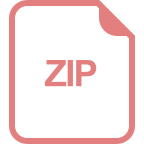
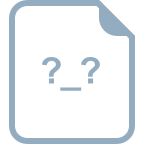