opencv只对图像中矩形区域做二值化处理C++例程
时间: 2024-02-01 13:13:43 浏览: 80
下面是一个使用 OpenCV 在图像中提取矩形区域并对其进行二值化处理的 C++ 例程:
```c++
#include <opencv2/opencv.hpp>
int main(int argc, char** argv)
{
// 读取图像
cv::Mat image = cv::imread("example.jpg");
// 灰度化
cv::Mat gray;
cv::cvtColor(image, gray, cv::COLOR_BGR2GRAY);
// 二值化
cv::Mat binary;
cv::threshold(gray, binary, 0, 255, cv::THRESH_BINARY | cv::THRESH_OTSU);
// 查找轮廓
std::vector<std::vector<cv::Point>> contours;
cv::findContours(binary, contours, cv::RETR_TREE, cv::CHAIN_APPROX_SIMPLE);
// 提取矩形区域
std::vector<cv::Rect> rects;
for (const auto& contour : contours) {
cv::Rect rect = cv::boundingRect(contour);
if (rect.width > 10 && rect.height > 10 && rect.width < image.cols && rect.height < image.rows) {
rects.push_back(rect);
}
}
// 对矩形区域进行二值化
for (const auto& rect : rects) {
cv::Mat roi = binary(rect);
cv::threshold(roi, roi, 0, 255, cv::THRESH_BINARY | cv::THRESH_OTSU);
}
// 显示结果
cv::imshow("image", image);
cv::imshow("binary", binary);
cv::waitKey(0);
return 0;
}
```
这个例程中,我们首先读取了一张图像并将其转换成灰度图像。然后,我们使用 Otsu 自适应阈值算法对图像进行二值化处理。接下来,我们使用 `findContours` 函数查找图像中的轮廓,并使用 `boundingRect` 函数提取矩形区域。最后,我们对矩形区域进行二值化处理,并显示结果。
阅读全文
相关推荐


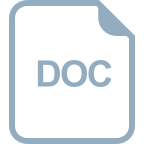
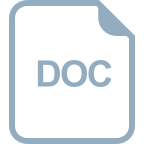
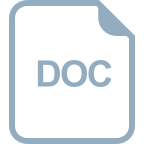
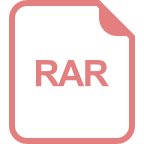
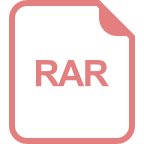
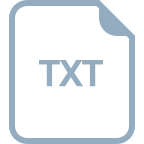
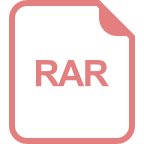
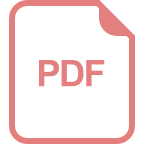
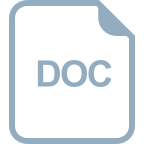
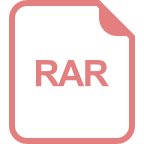
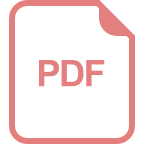