用opencv写一个图像处理hog特征提取
时间: 2023-11-14 22:01:47 浏览: 91
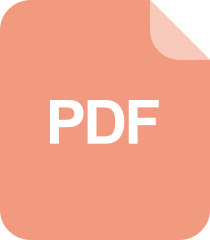
基于hog特征提取
好的,我可以帮助您用 OpenCV 编写一个图像处理程序来提取 HOG(方向梯度直方图)特征。 HOG 特征是一种用于对象检测的特征描述符,它被广泛应用于人脸识别、行人检测等领域。
以下是用 OpenCV 实现 HOG 特征提取的一些步骤:
1. 加载图像并转为灰度图像
```python
import cv2
# 加载图像
image = cv2.imread("input_image.jpg")
# 转为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
```
2. 计算梯度幅值和方向
```python
# 计算梯度幅值和方向
gx = cv2.Sobel(gray, cv2.CV_32F, 1, 0)
gy = cv2.Sobel(gray, cv2.CV_32F, 0, 1)
magnitude, angle = cv2.cartToPolar(gx, gy, angleInDegrees=True)
```
3. 划分图像为 cell,并计算每个 cell 内的方向直方图
```python
# 划分图像为 8x8 的 cell
cell_size = (8, 8)
blocks_per_cell = (1, 1)
cells_per_block = (2, 2)
# 计算每个 cell 内的方向直方图
histogram = cv2.HOGDescriptor(
_winSize=(gray.shape[1] // cell_size[1] * cell_size[1],
gray.shape[0] // cell_size[0] * cell_size[0]),
_blockSize=(cell_size[1] * cells_per_block[1],
cell_size[0] * cells_per_block[0]),
_blockStride=(cell_size[1], cell_size[0]),
_cellSize=(cell_size[1], cell_size[0]),
_nbins=9).compute(gray)
```
4. 将所有 cell 的方向直方图串联起来,形成最终的 HOG 特征描述符
```python
# 将所有 cell 的方向直方图串联起来
features = []
for i in range(histogram.shape[0] - cells_per_block[0] + 1):
for j in range(histogram.shape[1] - cells_per_block[1] + 1):
block_feature = []
for k in range(cells_per_block[0]):
for l in range(cells_per_block[1]):
cell_feature = histogram[i + k, j + l, :]
block_feature.extend(cell_feature)
norm = np.sqrt(np.sum(np.array(block_feature)**2) + 1e-6)
block_feature = [ele / norm for ele in block_feature]
features.extend(block_feature)
# 形成最终的 HOG 特征描述符
hog_descriptor = np.array(features)
```
以上是用 OpenCV 编写的 HOG 特征提取程序的基本过程,您可以在此基础上进行更详细的定制。
阅读全文
相关推荐
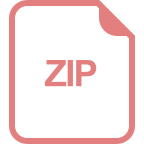
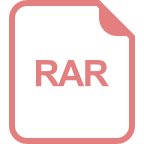
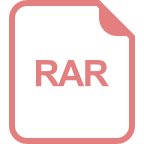
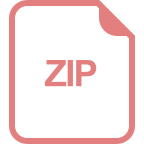
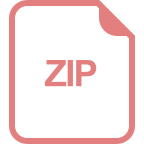
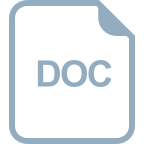
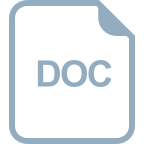
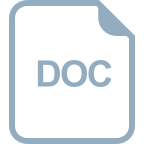
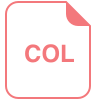

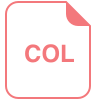
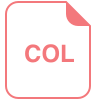
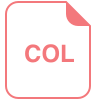