操作系统进程调度实验
时间: 2023-12-11 19:30:50 浏览: 105
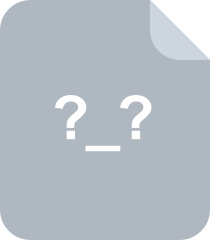
操作系统 进程调度实验
操作系统进程调度是操作系统中非常重要的一部分,它负责管理和调度系统中的进程,以便最大限度地利用系统资源并提高系统的性能。下面是一个简单的进程调度模拟程序的示例:
```python
# 定义进程类
class Process:
def __init__(self, pid, arrival_time, burst_time, priority):
self.pid = pid
self.arrival_time = arrival_time
self.burst_time = burst_time
self.priority = priority
self.waiting_time = 0
self.turnaround_time = 0
def __str__(self):
return f'Process {self.pid}'
# 先进先出调度算法
def fifo(processes):
current_time = 0
for process in processes:
if process.arrival_time > current_time:
current_time = process.arrival_time
process.waiting_time = current_time - process.arrival_time
process.turnaround_time = process.waiting_time + process.burst_time
current_time += process.burst_time
print(f'{process} 执行完毕,等待时间为 {process.waiting_time},周转时间为 {process.turnaround_time}')
# 基于优先数的调度算法
def priority(processes):
current_time = 0
while processes:
highest_priority = min(processes, key=lambda x: x.priority)
processes.remove(highest_priority)
if highest_priority.arrival_time > current_time:
current_time = highest_priority.arrival_time
highest_priority.waiting_time = current_time - highest_priority.arrival_time
highest_priority.turnaround_time = highest_priority.waiting_time + highest_priority.burst_time
current_time += highest_priority.burst_time
print(f'{highest_priority} 执行完毕,等待时间为 {highest_priority.waiting_time},周转时间为 {highest_priority.turnaround_time}')
# 最短执行时间调度算法
def sjf(processes):
current_time = 0
while processes:
shortest_job = min(processes, key=lambda x: x.burst_time)
processes.remove(shortest_job)
if shortest_job.arrival_time > current_time:
current_time = shortest_job.arrival_time
shortest_job.waiting_time = current_time - shortest_job.arrival_time
shortest_job.turnaround_time = shortest_job.waiting_time + shortest_job.burst_time
current_time += shortest_job.burst_time
print(f'{shortest_job} 执行完毕,等待时间为 {shortest_job.waiting_time},周转时间为 {shortest_job.turnaround_time}')
# 测试
if __name__ == '__main__':
processes = [
Process(1, 0, 5, 3),
Process(2, 1, 3, 1),
Process(3, 2, 8, 2),
Process(4, 3, 6, 4),
Process(5, 4, 1, 5),
Process(6, 5, 7, 6),
Process(7, 6, 2, 7),
Process(8, 7, 4, 8),
Process(9, 8, 3, 9),
Process(10, 9, 5, 10)
]
print('先进先出调度算法:')
fifo(processes.copy())
print('基于优先数的调度算法:')
priority(processes.copy())
print('最短执行时间调度算法:')
sjf(processes.copy())
```
阅读全文
相关推荐
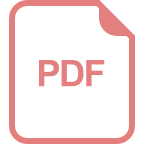
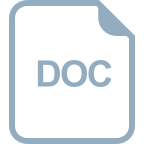















