9.1 使用多进程消息队列的方式写一个聊天程序。 有两个进程sendA和sendB。他们分别向recv进程发送不同类型的消息。进程recv接受消息前,都可以指定要获取的文件类型。
时间: 2024-02-28 10:56:34 浏览: 54
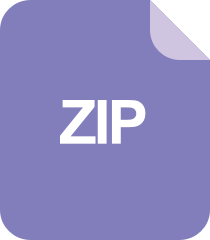
用消息队列,实现聊天程序

这里是一个使用多进程消息队列的方式写的聊天程序,包括两个发送进程sendA和sendB,以及一个接收进程recv。sendA和sendB向recv进程发送不同类型的消息,recv进程可以指定要获取的消息类型。
sendA进程的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSG_TYPE_A 1
struct msgbuf {
long mtype;
char mtext[256];
};
int main() {
int msgid;
struct msgbuf msg;
// 创建或获取消息队列
msgid = msgget(1234, IPC_CREAT | 0666);
if (msgid == -1) {
perror("msgget");
exit(1);
}
// 发送消息
msg.mtype = MSG_TYPE_A;
while (1) {
printf("sendA: ");
fgets(msg.mtext, sizeof(msg.mtext), stdin);
if (msgsnd(msgid, &msg, strlen(msg.mtext) + 1, 0) == -1) {
perror("msgsnd");
break;
}
}
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(1);
}
return 0;
}
```
sendB进程的代码与sendA进程类似,只需要修改MSG_TYPE_A为MSG_TYPE_B即可。
recv进程的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSG_TYPE_A 1
#define MSG_TYPE_B 2
struct msgbuf {
long mtype;
char mtext[256];
};
int main() {
int msgid;
struct msgbuf msg;
// 创建或获取消息队列
msgid = msgget(1234, IPC_CREAT | 0666);
if (msgid == -1) {
perror("msgget");
exit(1);
}
// 接收消息
while (1) {
printf("recv: ");
fgets(msg.mtext, sizeof(msg.mtext), stdin);
if (strcmp(msg.mtext, "A\n") == 0) {
// 接收类型为MSG_TYPE_A的消息
if (msgrcv(msgid, &msg, sizeof(msg.mtext), MSG_TYPE_A, 0) == -1) {
perror("msgrcv");
break;
}
printf("recvA: %s", msg.mtext);
} else if (strcmp(msg.mtext, "B\n") == 0) {
// 接收类型为MSG_TYPE_B的消息
if (msgrcv(msgid, &msg, sizeof(msg.mtext), MSG_TYPE_B, 0) == -1) {
perror("msgrcv");
break;
}
printf("recvB: %s", msg.mtext);
} else {
printf("unknown message type\n");
}
}
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(1);
}
return 0;
}
```
使用方法:先运行recv进程,之后分别运行sendA和sendB进程,根据提示输入要发送的消息内容即可。在recv进程中,输入A可以接收类型为MSG_TYPE_A的消息,输入B可以接收类型为MSG_TYPE_B的消息。
阅读全文
相关推荐
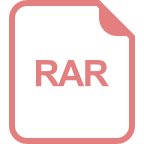
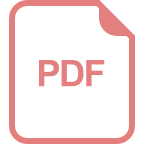
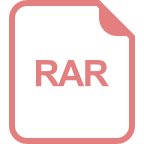
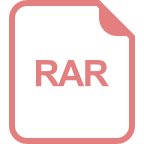
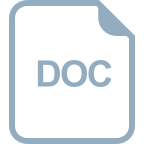
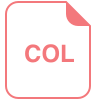

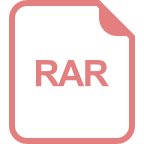
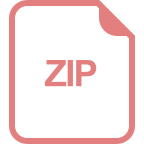