使用QProcess运行一条linux命令
时间: 2023-11-08 22:06:04 浏览: 44
Sure, 可以使用QProcess在Qt中运行一条linux命令。以下是示例代码:
```
QProcess process;
process.start("ls");
process.waitForFinished();
QString output = process.readAllStandardOutput();
QString error = process.readAllStandardError();
```
这将运行'ls'命令,并返回该命令的输出和错误(如果有的话)。如果你需要运行其他命令,请直接替换'ls'即可。
相关问题
QProcess执行多个linux命令
在Qt中,可以使用QProcess类来执行多个Linux命令。下面是一个示例代码:
```
QProcess process;
QStringList commands;
commands << "ls" << "pwd" << "echo hello";
foreach (QString command, commands) {
process.start(command);
process.waitForFinished(-1); // 等待进程执行完毕
QByteArray output = process.readAllStandardOutput(); // 获取命令行的输出结果
qDebug() << "Command:" << command;
qDebug() << "Output:" << output;
}
```
在这个示例中,我们创建了一个QProcess对象,并使用QStringList来存储要执行的多个命令。然后,通过foreach循环遍历每个命令,并使用QProcess的start方法执行命令。接着,我们使用waitForFinished方法等待进程执行完毕,并使用readAllStandardOutput方法获取命令行的输出结果。最后,我们打印出每个命令和对应的输出结果。
值得注意的是,waitForFinished方法的参数-1表示等待进程执行完毕,而readAllStandardOutput方法用于获取命令行的输出结果。你可以根据自己的需求来调整这些方法的参数和使用方式。
QProcess 执行linux的sudo命令
可以使用`QProcess`执行Linux的`sudo`命令。需要注意的是,使用`sudo`命令需要输入密码,因此需要通过`QProcess`的`write`方法将密码输入到命令行中。
以下是一个示例代码:
```cpp
#include <QCoreApplication>
#include <QProcess>
#include <QDebug>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
QString command = "sudo ls /root"; // 需要使用sudo执行的命令
QProcess process;
process.start(command);
process.waitForStarted();
// 输入密码
QString password = "your_password";
process.write((password + "\n").toUtf8());
process.waitForBytesWritten();
process.waitForFinished();
qDebug() << process.readAllStandardOutput();
return a.exec();
}
```
在上面的代码中,我们使用`QProcess`执行了一个`sudo ls /root`命令,并且通过`write`方法将密码输入到了命令行中。最后,我们通过`readAllStandardOutput`方法获取了命令的输出结果。
相关推荐
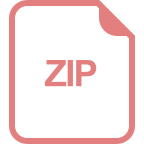
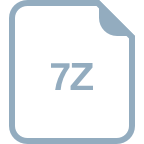












