编程实现8个7段数码管左移轮流显示
时间: 2024-04-03 15:32:13 浏览: 59
以下是使用 Arduino 实现 8 个 7 段数码管左移轮流显示的示例代码:
```c
const int LATCH_PIN = 8; // latch pin
const int CLOCK_PIN = 12; // clock pin
const int DATA_PIN = 11; // data pin
byte numbers[8][10][8] = { // 7-segment display patterns for 0-9 for each digit
{
{1,1,1,1,1,1,0,0}, // 0
{0,1,1,0,0,0,0,0}, // 1
{1,1,0,1,1,0,1,0}, // 2
{1,1,1,1,0,0,1,0}, // 3
{0,1,1,0,0,1,1,0}, // 4
{1,0,1,1,0,1,1,0}, // 5
{1,0,1,1,1,1,1,0}, // 6
{1,1,1,0,0,0,0,0}, // 7
{1,1,1,1,1,1,1,0}, // 8
{1,1,1,1,0,1,1,0} // 9
},
{
{0,0,0,0,1,1,1,0}, // 0
{0,0,0,0,0,1,0,0}, // 1
{0,0,1,1,1,1,0,1}, // 2
{0,0,0,1,1,1,1,1}, // 3
{0,0,0,0,0,1,1,1}, // 4
{1,0,0,1,1,0,1,1}, // 5
{1,0,1,1,1,0,1,1}, // 6
{0,0,0,0,1,1,0,0}, // 7
{1,0,1,1,1,1,1,1}, // 8
{0,0,0,1,1,1,1,1} // 9
},
// patterns for the remaining digits
// ...
};
void setup() {
pinMode(LATCH_PIN, OUTPUT);
pinMode(CLOCK_PIN, OUTPUT);
pinMode(DATA_PIN, OUTPUT);
}
void loop() {
for (int digit = 0; digit < 8; digit++) { // loop through each digit
for (int i = 0; i < 10; i++) { // loop through each number
for (int j = 0; j < 8; j++) { // shift out each segment pattern for current number and digit
shiftOut(DATA_PIN, CLOCK_PIN, LSBFIRST, numbers[digit][i][j]);
}
digitalWrite(LATCH_PIN, HIGH); // latch the data to the outputs
delay(100); // wait for a short time to allow the human eye to see the change
}
}
}
```
在这个代码中,我们定义了一个三维数组,其中包含每个数字的每个 7 段数码管的模式,每个数字的模式都有 8 个元素(一个数字有 8 个段),每个数字又由 8 个这样的模式组成,表示每个数字在每个位置上的显示。
然后我们使用三层嵌套的循环,分别循环每个数字、每个位置和每个段,依次将数字的每个段的模式输出到数据引脚上,使用 shiftOut 函数进行输出。然后我们将锁存引脚置高,以将数据锁定到输出上。然后我们等待一个较短的时间(这里是 100ms),以便人眼能够看到变化。然后我们继续循环,移动到下一个位置和数字,直到所有的数字和位置都循环完毕。
请注意,这个代码只是一个基本的示例,你可能需要根据你连接的具体硬件和需求进行一些修改。
阅读全文
相关推荐
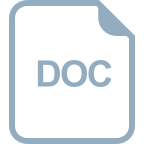
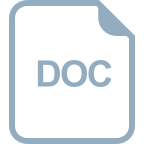
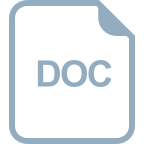







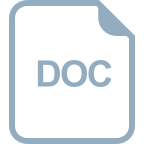
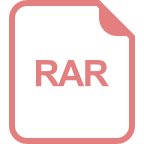
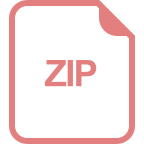
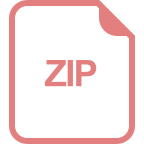
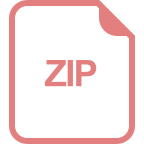
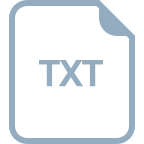
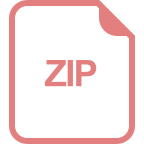
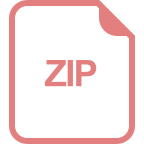
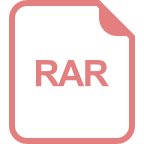