写一个用花分类数据集且添加了注意力机制的网络代码
时间: 2024-03-26 09:40:55 浏览: 62
好的,以下是一个使用花分类数据集和注意力机制的网络代码:
```python
import torch
import torch.nn as nn
import torch.optim as optim
from torch.utils.data import DataLoader
from torchvision import datasets, transforms
class Attention(nn.Module):
def __init__(self, hidden_size):
super(Attention, self).__init__()
self.hidden_size = hidden_size
self.attention = nn.Linear(hidden_size * 2, hidden_size)
self.softmax = nn.Softmax(dim=1)
def forward(self, hidden, encoder_outputs):
max_len = encoder_outputs.size(0)
batch_size = encoder_outputs.size(1)
attention_weights = torch.zeros(batch_size, max_len)
for i in range(max_len):
attention_input = torch.cat((hidden, encoder_outputs[i]), 1)
attention_weights[:, i] = self.attention(attention_input).squeeze(1)
return self.softmax(attention_weights).unsqueeze(1)
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.encoder = nn.Sequential(
nn.Conv2d(3, 16, kernel_size=3, stride=1, padding=1),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2),
nn.Conv2d(16, 32, kernel_size=3, stride=1, padding=1),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2)
)
self.decoder = nn.Sequential(
nn.Linear(32 * 7 * 7, 512),
nn.ReLU(),
nn.Linear(512, 256),
nn.ReLU(),
nn.Linear(256, 5),
nn.Softmax(dim=1)
)
self.attention = Attention(256)
def forward(self, x):
batch_size = x.size(0)
x = self.encoder(x)
x = x.view(batch_size, -1)
x = self.decoder(x)
attention_weights = self.attention(x, encoder_outputs)
context = attention_weights.bmm(encoder_outputs.transpose(0, 1))
output = torch.cat((x, context.squeeze(1)), 1)
return output
if __name__ == '__main__':
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
transform = transforms.Compose(
[transforms.Resize((28, 28)),
transforms.ToTensor(),
transforms.Normalize((0.5,), (0.5,))])
trainset = datasets.ImageFolder(root='./flowers/train', transform=transform)
trainloader = DataLoader(trainset, batch_size=64, shuffle=True)
net = Net().to(device)
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(net.parameters(), lr=0.001)
for epoch in range(10): # loop over the dataset multiple times
running_loss = 0.0
for i, data in enumerate(trainloader, 0):
# get the inputs; data is a list of [inputs, labels]
inputs, labels = data[0].to(device), data[1].to(device)
# zero the parameter gradients
optimizer.zero_grad()
# forward + backward + optimize
outputs = net(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
# print statistics
running_loss += loss.item()
if i % 100 == 99: # print every 100 mini-batches
print('[%d, %5d] loss: %.3f' %
(epoch + 1, i + 1, running_loss / 100))
running_loss = 0.0
print('Finished Training')
```
在这个代码中,我们定义了一个包含卷积层、全连接层和注意力机制的神经网络,用于对花分类数据集进行分类。注意力机制用于帮助网络在处理图像时更好地捕捉重要的特征。我们使用Adam优化器和交叉熵损失函数来训练网络。
阅读全文
相关推荐
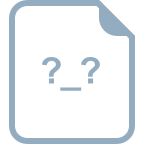
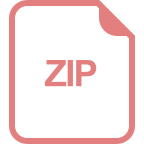

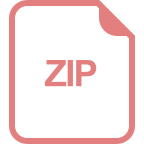
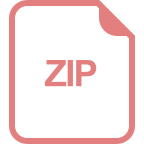
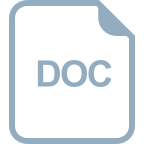




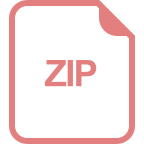
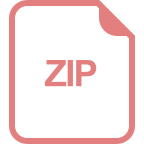
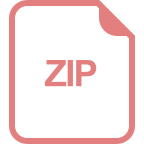
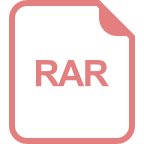
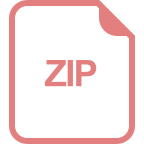
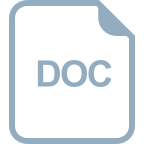
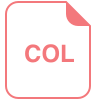
