超市管理系统C++
时间: 2023-07-10 20:06:01 浏览: 60
超市管理系统是一个非常复杂的软件系统,需要考虑多种因素,包括库存管理、销售统计、会员管理、收银管理等。以下是一个简单的超市管理系统的C++代码示例,仅供参考:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Product {
private:
string name;
double price;
int count;
public:
Product(string n, double p, int c) {
name = n;
price = p;
count = c;
}
string getName() {
return name;
}
double getPrice() {
return price;
}
int getCount() {
return count;
}
void setCount(int c) {
count = c;
}
void print() {
cout << name << "\t" << price << "\t" << count << endl;
}
};
class Cart {
private:
vector<Product> products;
public:
void add(Product p) {
products.push_back(p);
}
double getTotalPrice() {
double total = 0;
for (int i = 0; i < products.size(); i++) {
total += products[i].getPrice();
}
return total;
}
void print() {
for (int i = 0; i < products.size(); i++) {
products[i].print();
}
cout << "Total: " << getTotalPrice() << endl;
}
};
class Inventory {
private:
vector<Product> products;
public:
void add(Product p) {
products.push_back(p);
}
void print() {
for (int i = 0; i < products.size(); i++) {
products[i].print();
}
}
Product find(string name) {
for (int i = 0; i < products.size(); i++) {
if (products[i].getName() == name) {
return products[i];
}
}
return Product("", 0, 0);
}
void update(string name, int count) {
for (int i = 0; i < products.size(); i++) {
if (products[i].getName() == name) {
products[i].setCount(count);
break;
}
}
}
};
class Sale {
private:
Cart cart;
double discount;
public:
Sale(Cart c, double d) {
cart = c;
discount = d;
}
double getTotalPrice() {
return cart.getTotalPrice() * (1 - discount);
}
void print() {
cart.print();
cout << "Discount: " << discount << endl;
cout << "Total: " << getTotalPrice() << endl;
}
};
class Member {
private:
string name;
double balance;
double discount;
public:
Member(string n, double b, double d) {
name = n;
balance = b;
discount = d;
}
string getName() {
return name;
}
double getBalance() {
return balance;
}
double getDiscount() {
return discount;
}
void setBalance(double b) {
balance = b;
}
};
class Membership {
private:
vector<Member> members;
public:
void add(Member m) {
members.push_back(m);
}
Member find(string name) {
for (int i = 0; i < members.size(); i++) {
if (members[i].getName() == name) {
return members[i];
}
}
return Member("", 0, 0);
}
void updateBalance(string name, double amount) {
for (int i = 0; i < members.size(); i++) {
if (members[i].getName() == name) {
members[i].setBalance(members[i].getBalance() + amount);
break;
}
}
}
};
int main() {
Inventory inventory;
inventory.add(Product("Apple", 1.0, 10));
inventory.add(Product("Banana", 2.0, 20));
inventory.add(Product("Orange", 3.0, 30));
Membership membership;
membership.add(Member("John", 0, 0.1));
membership.add(Member("Mary", 100, 0.2));
Cart cart;
cart.add(inventory.find("Apple"));
cart.add(inventory.find("Banana"));
double discount = 0;
string name;
cout << "Are you a member? (Y/N) ";
char answer;
cin >> answer;
if (answer == 'Y' || answer == 'y') {
cout << "Please enter your name: ";
cin >> name;
Member member = membership.find(name);
if (member.getName() == "") {
cout << "Member not found." << endl;
} else {
cout << "Welcome, " << member.getName() << "." << endl;
discount = member.getDiscount();
double balance = member.getBalance();
if (balance > 0) {
cout << "You have a balance of " << balance << "." << endl;
cout << "Do you want to use it to pay for this purchase? (Y/N) ";
char answer;
cin >> answer;
if (answer == 'Y' || answer == 'y') {
if (balance >= cart.getTotalPrice()) {
cout << "Payment successful." << endl;
membership.updateBalance(name, -cart.getTotalPrice());
return 0;
} else {
cout << "Insufficient balance." << endl;
}
}
}
}
}
Sale sale(cart, discount);
sale.print();
cout << "Enter the amount paid: ";
double paid;
cin >> paid;
if (paid >= sale.getTotalPrice()) {
cout << "Change: " << paid - sale.getTotalPrice() << endl;
if (name != "") {
membership.updateBalance(name, sale.getTotalPrice() * (1 - discount));
}
for (int i = 0; i < cart.getCount(); i++) {
Product product = cart.getProduct(i);
inventory.update(product.getName(), product.getCount() - 1);
}
} else {
cout << "Insufficient payment." << endl;
}
return 0;
}
```
注意,这只是一个简单的示例,实际的超市管理系统需要更复杂的功能和更完善的界面设计。
相关推荐











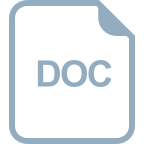