c语言ping程序代码
时间: 2023-11-07 10:55:17 浏览: 94
下面是一个简单的 C 语言 Ping 程序,可以用于在 Windows 系统下测试网络连接:
```
#include <stdio.h>
#include <winsock2.h>
#include <windows.h>
#include <iphlpapi.h>
#include <icmpapi.h>
#pragma comment(lib, "iphlpapi.lib")
#pragma comment(lib, "ws2_32.lib")
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Usage: %s <host>\n", argv[0]);
return 1;
}
const char* host = argv[1];
if (strlen(host) > MAX_PATH) {
printf("Host name is too long!\n");
return 1;
}
WSADATA wsaData;
if (WSAStartup(MAKEWORD(2, 2), &wsaData) != 0) {
printf("WSAStartup failed!\n");
return 1;
}
HANDLE icmpHandle = IcmpCreateFile();
if (icmpHandle == INVALID_HANDLE_VALUE) {
printf("IcmpCreateFile failed!\n");
WSACleanup();
return 1;
}
char sendBuf[32] = { 0 };
DWORD dataSize = sizeof(sendBuf);
DWORD ipAddr = inet_addr(host);
if (ipAddr == INADDR_NONE) {
struct hostent* hostEnt = gethostbyname(host);
if (hostEnt == NULL || hostEnt->h_addrtype != AF_INET) {
printf("Unknown host %s!\n", host);
IcmpCloseHandle(icmpHandle);
WSACleanup();
return 1;
}
ipAddr = *(DWORD*)hostEnt->h_addr_list[0];
}
printf("Pinging %s [%s] with %d bytes of data:\n", host, inet_ntoa(*(in_addr*)&ipAddr), dataSize);
for (int i = 0; i < 4; i++) {
if (IcmpSendEcho(icmpHandle, ipAddr, sendBuf, dataSize, NULL, NULL, 1000) == 0) {
printf("Request timed out.\n");
} else {
printf("Reply from %s: %d bytes in %ldms TTL=%d\n",
inet_ntoa(*(in_addr*)&ipAddr), dataSize,
((PIP_ECHO_REPLY)sendBuf)->RoundTripTime,
((PIP_ECHO_REPLY)sendBuf)->Options.Ttl);
}
Sleep(1000);
}
IcmpCloseHandle(icmpHandle);
WSACleanup();
return 0;
}
```
这个程序使用了 WinSock 和 ICMP 协议,可以在 Windows 系统下运行。它会发送四个 ICMP 回显请求(即 Ping 请求),并等待每个请求的回复。如果在一定时间内没有收到回复,就认为该请求超时。如果收到回复,则打印回复报文的信息,包括源 IP 地址、数据长度、往返时间(即时延)和 TTL(生存时间)。
阅读全文
相关推荐
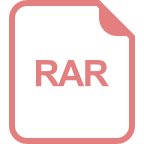
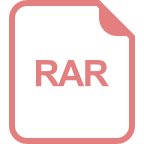
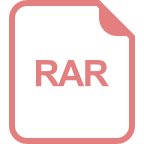
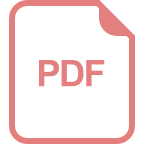
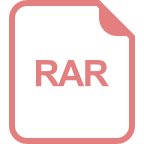
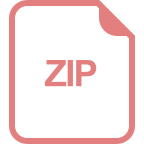
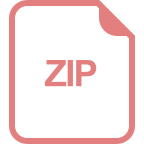
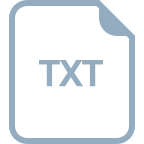
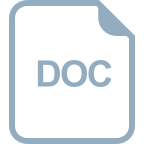
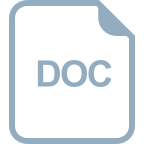
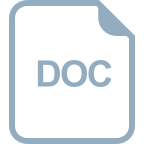
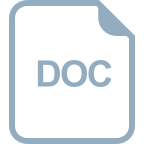
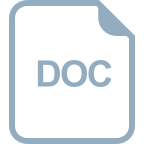
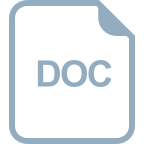