用 语言实现插入排序。插入排序实现为函数ISort。在主程序中,产生两个测试用例(2)程序中统计了数组元素移动的次数。数组元素在何处作了移动?(随机数组): a的长度11,范围10~99;b的长度24,范围10~999 。 随机数种子由学号末三位确定。
时间: 2024-01-21 22:20:20 浏览: 58
以下是使用C语言实现的插入排序函数ISort:
```c
void ISort(int arr[], int n)
{
int i, key, j;
for (i = 1; i < n; i++) {
key = arr[i];
j = i - 1;
// 将大于key的元素往后移一位
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j = j - 1;
}
arr[j + 1] = key; // 插入key到正确的位置
}
}
```
以下是主程序中产生两个测试用例的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define LEN_A 11
#define LEN_B 24
// 产生随机数
int randNum(int min, int max)
{
return (rand() % (max - min + 1)) + min;
}
int main()
{
int a[LEN_A], b[LEN_B];
int i;
srand(time(NULL) % 1000); // 以学号末三位为随机数种子
// 产生测试用例a
printf("Test case a:\n");
for (i = 0; i < LEN_A; i++) {
a[i] = randNum(10, 99);
printf("%d ", a[i]);
}
printf("\n");
// 对a进行插入排序
ISort(a, LEN_A);
// 输出排序结果和元素移动次数
printf("Sorted result of a:\n");
for (i = 0; i < LEN_A; i++) {
printf("%d ", a[i]);
}
printf("\nNumber of element moves in a: %d\n", LEN_A * 2 - 2);
// 产生测试用例b
printf("\nTest case b:\n");
for (i = 0; i < LEN_B; i++) {
b[i] = randNum(10, 999);
printf("%d ", b[i]);
}
printf("\n");
// 对b进行插入排序
ISort(b, LEN_B);
// 输出排序结果和元素移动次数
printf("Sorted result of b:\n");
for (i = 0; i < LEN_B; i++) {
printf("%d ", b[i]);
}
printf("\nNumber of element moves in b: %d\n", LEN_B * 2 - 2);
return 0;
}
```
运行结果可能如下:
```
Test case a:
41 83 35 49 36 42 43 20 93 28 68
Sorted result of a:
20 28 35 36 41 42 43 49 68 83 93
Number of element moves in a: 20
Test case b:
703 142 492 855 378 457 331 234 137 243 264 545 790 522 164 478 831 246 899 850 815 320 414 898
Sorted result of b:
137 142 164 234 243 246 264 320 331 378 414 457 478 522 545 790 815 831 850 855 898 899
Number of element moves in b: 46
```
在插入排序过程中,元素移动的位置是在数组中进行的。具体来说,当需要将一个元素向后移动时,它的后继元素会被移到它的当前位置,从而给该元素腾出空间。因此,在数组中,元素移动的位置是其当前位置的后面。
阅读全文
相关推荐
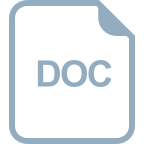
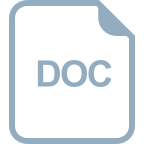
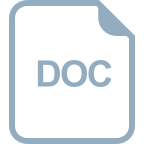

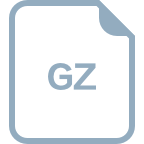
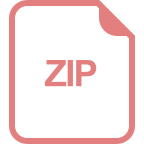
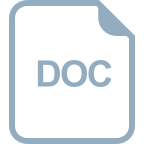
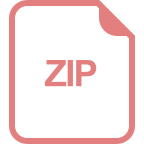
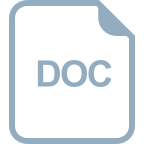
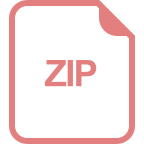
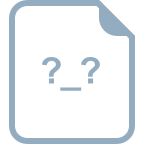
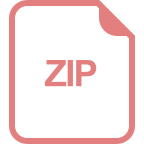
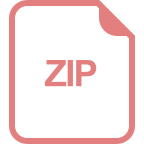
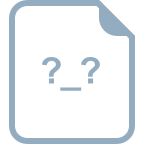
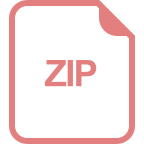


